As software developers, we often hear about the importance of SOLID principles. But how do these principles translate into real-world applications? In this post, we’ll explore concrete examples of SOLID principles in action, demonstrating how they can lead to more maintainable and scalable codebases.
Let’s dive into each principle with practical scenarios that you might encounter in your day-to-day development work.
Introduction
The SOLID principles are a foundational set of five design principles in object-orien- ted programming that promote the development of high-quality, maintainable, and scalable software applications. Formulated by Robert C. Martin in the early 2000s, these principles—Single Responsibility, Open/Closed, Liskov Substitution, Interface Segregation, and Dependency Inversion—have gained widespread adoption among software developers and engineers as essential guidelines for creating robust and adaptable codebases. Their relevance is underscored by the increasing complexity of software systems in modern development practices, making adherence to these principles crucial for effective coding and system architecture.[1][2][3].
Each principle addresses specific aspects of software design, contributing to im- proved maintainability, extensibility, and testability. For instance, the Single Respon- sibility Principle advocates that a class should focus on a single task, while the Open/Closed Principle suggests that classes should be open for extension yet closed for modification. These guidelines collectively enhance code organization and reduce the risk of bugs arising from changes, promoting a more efficient development lifecycle and better collaboration among teams.[4][5][6].
Despite their benefits, the application of SOLID principles is not without challenges. Developers often struggle with common pitfalls, such as over-segregation or un- der-segregation of interfaces, which can complicate the codebase rather than simplify it. Furthermore, violations of the Liskov Substitution Principle can lead to unexpected behaviors, highlighting the importance of careful design and adherence to these principles in practice. Continuous education and practical application of the SOLID principles are vital for software teams aiming to create high-quality, maintainable code that can adapt to changing requirements.[7][8][9].
In summary, the SOLID principles serve as a critical framework for software develop- ment, addressing fundamental issues related to code quality and system reliability. Their widespread recognition and application in the industry underscore their signifi- cance in fostering effective design practices that ultimately lead to more resilient and manageable software solutions.[10][11][12].
Single Responsibility Principle (SRP)
The Single Responsibility Principle states that a class should have only one reason to change. In other words, a class should have a single, well-defined purpose.
Real-world example: Consider a user management system. Initially, you might have a User class that handles user data, authentication, and email notifications:
public class User
{
public void Register(string username, string password)
{
// Registration logic
}
public bool Authenticate(string username, string password)
{
// Authentication logic
}
public void SendWelcomeEmail(string email)
{
// Email sending logic
}
}
This violates SRP because the User class is responsible for multiple concerns. Let’s refactor this to adhere to SRP:
public class User
{
public string Username { get; set; }
public string Password { get; set; }
}
public class UserAuthenticator
{
public bool Authenticate(User user, string password)
{
// Authentication logic
}
}
public class EmailService
{
public void SendWelcomeEmail(string email)
{
// Email sending logic
}
}
Now, each class has a single responsibility, making the code more modular and easier to maintain.
Open-Closed Principle (OCP)
The Open-Closed Principle states that software entities should be open for extension but closed for modification. This means we should be able to add new functionality without changing existing code.
Real-world example: Let’s say we’re building a payment processing system for an e-commerce platform:
public class PaymentProcessor
{
public void ProcessPayment(string paymentMethod, decimal amount)
{
if (paymentMethod == "CreditCard")
{
// Process credit card payment
}
else if (paymentMethod == "PayPal")
{
// Process PayPal payment
}
}
}
This design violates OCP because adding a new payment method requires modifying the existing PaymentProcessor class. Let’s refactor this to follow OCP:
public interface IPaymentMethod
{
void ProcessPayment(decimal amount);
}
public class CreditCardPayment : IPaymentMethod
{
public void ProcessPayment(decimal amount)
{
// Process credit card payment
}
}
public class PayPalPayment : IPaymentMethod
{
public void ProcessPayment(decimal amount)
{
// Process PayPal payment
}
}
public class PaymentProcessor
{
public void ProcessPayment(IPaymentMethod paymentMethod, decimal amount)
{
paymentMethod.ProcessPayment(amount);
}
}
Now, we can easily add new payment methods by creating new classes that implement IPaymentMethod, without modifying the existing PaymentProcessor class.
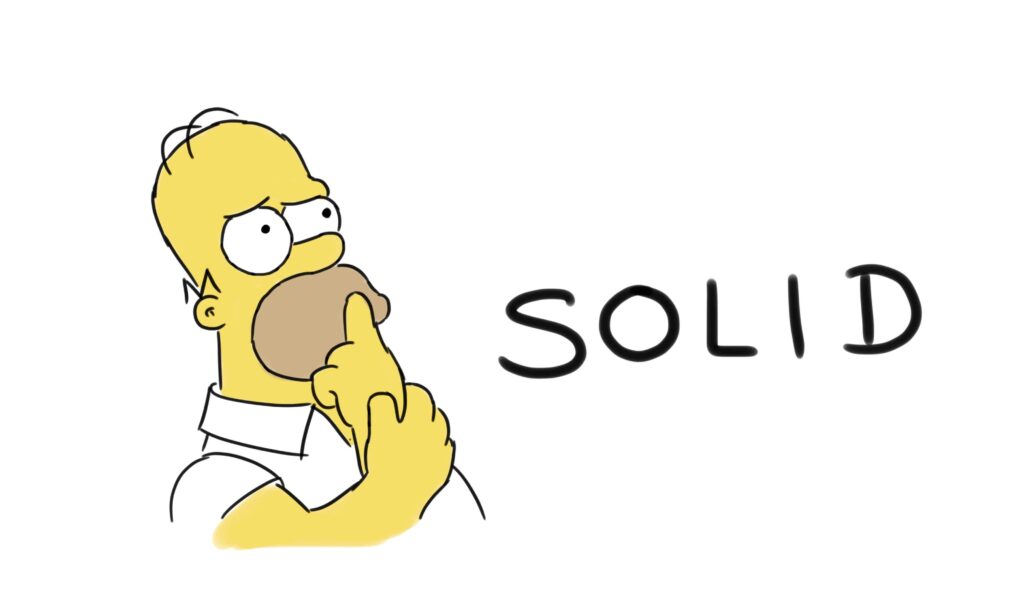
Liskov Substitution Principle (LSP)
The Liskov Substitution Principle states that objects of a superclass should be replaceable with objects of its subclasses without affecting the correctness of the program.
Real-world example: Consider a shape hierarchy in a drawing application:
public class Rectangle
{
public virtual void SetWidth(double width) { /* ... */ }
public virtual void SetHeight(double height) { /* ... */ }
public double GetArea() { /* ... */ }
}
public class Square : Rectangle
{
public override void SetWidth(double width)
{
base.SetWidth(width);
base.SetHeight(width);
}
public override void SetHeight(double height)
{
base.SetHeight(height);
base.SetWidth(height);
}
}
This violates LSP because a Square is not substitutable for a Rectangle. If we expect a Rectangle but get a Square, the behavior of SetWidth and SetHeight will be unexpected. Let’s refactor this to adhere to LSP:
public interface IShape
{
double GetArea();
}
public class Rectangle : IShape
{
public double Width { get; set; }
public double Height { get; set; }
public double GetArea() => Width * Height;
}
public class Square : IShape
{
public double SideLength { get; set; }
public double GetArea() => SideLength * SideLength;
}
Now, both Rectangle and Square implement the IShape interface, and we can use them interchangeably when we only need to calculate the area.
Interface Segregation Principle (ISP)
The Interface Segregation Principle states that clients should not be forced to depend on interfaces they do not use. In other words, it’s better to have many smaller, specific interfaces rather than a few large, general-purpose ones.
Real-world example: Consider a multi-function printer interface:
public interface IPrinter
{
void Print(Document d);
void Scan(Document d);
void Fax(Document d);
void PhotoCopy(Document d);
}
This interface violates ISP because not all printers support all these functions. Let’s refactor this to adhere to ISP:
public interface IPrinter
{
void Print(Document d);
}
public interface IScanner
{
void Scan(Document d);
}
public interface IFaxMachine
{
void Fax(Document d);
}
public interface IPhotoCopier
{
void PhotoCopy(Document d);
}
public class AllInOnePrinter : IPrinter, IScanner, IFaxMachine, IPhotoCopier
{
// Implement all methods
}
public class SimplePrinter : IPrinter
{
// Implement only Print method
}
Now, clients can depend only on the interfaces they need, and we can create different types of printers with varying capabilities.
Dependency Inversion Principle (DIP)
The Dependency Inversion Principle states that high-level modules should not depend on low-level modules. Both should depend on abstractions. Additionally, abstractions should not depend on details; details should depend on abstractions.
Real-world example: Let’s consider a notification system in a social media application:
public class EmailNotifier
{
public void SendNotification(string message)
{
// Send email notification
}
}
public class NotificationService
{
private EmailNotifier _emailNotifier = new EmailNotifier();
public void Notify(string message)
{
_emailNotifier.SendNotification(message);
}
}
This violates DIP because the high-level NotificationService depends directly on the low-level EmailNotifier. Let’s refactor this to adhere to DIP:
public interface INotifier
{
void SendNotification(string message);
}
public class EmailNotifier : INotifier
{
public void SendNotification(string message)
{
// Send email notification
}
}
public class SMSNotifier : INotifier
{
public void SendNotification(string message)
{
// Send SMS notification
}
}
public class NotificationService
{
private readonly INotifier _notifier;
public NotificationService(INotifier notifier)
{
_notifier = notifier;
}
public void Notify(string message)
{
_notifier.SendNotification(message);
}
}
Now, the NotificationService depends on the INotifier abstraction, not on concrete implementations. This makes it easy to add new notification methods or change the existing ones without modifying the NotificationService class.
The SOLID Principles
The SOLID principles are a set of five design principles that aim to create high-quality, maintainable, and scalable software applications. Introduced by Robert C. Martin (often referred to as Uncle Bob) in the early 2000s, these principles have become
a cornerstone of good software design in the industry.
Single Responsibility Principle (SRP)
The Single Responsibility Principle asserts that a class should have only one reason to change, meaning it should focus on a single responsibility or task. This principle promotes modularity and makes the codebase easier to maintain and extend, as changes to one responsibility will not inadvertently affect others[1][2][3].
Open/Closed Principle (OCP)
The Open/Closed Principle states that software entities such as classes and modules should be open for extension but closed for modification. This allows developers to add new functionality without altering existing code, reducing the risk of introducing bugs and making the software more adaptable to change[1][4].
Liskov Substitution Principle (LSP)
The Liskov Substitution Principle emphasizes that objects of a superclass should be replaceable with objects of a subclass without affecting the correctness of the program. This principle ensures that derived classes extend the behavior of the base class without altering its fundamental characteristics, promoting reliability and flexibility[2][5].
Interface Segregation Principle (ISP)
The Interface Segregation Principle advocates for creating smaller, specific interfaces rather than a large, general-purpose interface. This principle encourages developers to design systems that do not force classes to implement methods they do not use, enhancing code clarity and reducing the impact of changes[2][5].
Dependency Inversion Principle (DIP)
The Dependency Inversion Principle asserts that high-level modules should not depend on low-level modules, but both should depend on abstractions. This principle
facilitates the decoupling of software components, making the code more flexible, easier to test, and simpler to manage in the long term[2][4][5].
By applying the SOLID principles, developers can create code that is well-organized, easier to understand, and more robust against future changes, thereby enhancing collaboration and efficiency in software development projects[6][3].
Transforming Your Code
Transforming your code involves applying design principles that enhance its maintain- ability, scalability, and overall quality. The SOLID principles, a set of five guidelines, play a critical role in this transformation by addressing common code issues and promoting best practices in software design.
Code Quality Challenges
Poorly structured code often results in complications such as tangled dependencies, repeated code, and difficulties in testing. These issues manifest as hidden problems where fixing one bug inadvertently introduces others, complicating the debugging process. The result is a codebase that is hard to understand and maintain, leading to the necessity of exhaustive testing with each modification made[7].
To mitigate these challenges, it is essential to adhere to the SOLID principles, which focus on reducing code complexity and promoting modularity. By following these principles, developers can create more flexible and manageable software that is easier to understand and fully tested[7][8].
Understanding SOLID Principles
The SOLID principles consist of five key tenets:
Single Responsibility Principle (SRP): This principle states that each class should have a single responsibility, leading to a more organized and streamlined codebase. By applying SRP, developers can create specialized components that are easier to maintain and adapt to future changes[9].
Open-Closed Principle (OCP): According to OCP, classes should be open for exten- sion but closed for modification. This means new functionality can be added without altering existing code, much like building onto a LEGO structure. Adhering to this principle prevents the introduction of bugs in stable systems and enhances code readability[9][8].
Liskov Substitution Principle (LSP): Objects of a superclass should be replaceable with objects of its subclasses without altering the correctness of the program. This principle ensures that derived classes extend the behavior of base classes in a meaningful way, enhancing code reliability[7].
Interface Segregation Principle (ISP): This principle advises against large interfaces that may require implementing unused methods. Instead, it encourages the creation of smaller, specific interfaces to increase cohesion and support the SRP. Adhering
to ISP can significantly reduce the complexity of code and make it easier to manage dependencies[10][11].
Dependency Inversion Principle (DIP): Higher-level modules should not depend on lower-level modules; both should depend on abstractions. This principle promotes loose coupling and makes the system more adaptable to change[12].
Real-World Application
Transforming code using the SOLID principles can lead to significant improvements. For instance, consider the Factory Method design pattern, which aids in object construction by determining the object type based on the requesting object. This decouples code dependencies, allowing for more flexible adaptations to changes, such as accommodating various database types without extensive modifications to the existing codebase[13][9].
Additionally, dynamic command discovery and auto-registration techniques can fully comply with the OCP, allowing new commands to be added without modifying existing code. This ensures that software remains stable and maintains its functionality while evolving to meet new requirements[14].
By continually revisiting and refining your code to align with the SOLID principles, you can enhance the maintainability, scalability, and reusability of your software, ultimately leading to a more robust architecture that is prepared for future challenges- [8][10].
Case Studies
E-commerce Platform Refactoring
In a medium-sized e-commerce platform, frequent issues arose within its product and order management services. These services were overly complex, handling various tasks such as database interactions, payment processing, and notifications. The challenge was that the codebase became hard to maintain and extend, leading to slow feature implementation and new bugs emerging with fixes[15].
Solution Implementation
The development team decided to refactor their NestJS services by applying the Single Responsibility Principle (SRP). They broke down the monolithic services into smaller, focused ones, creating separate services for payment processing, notifi- cations, and other functionalities[15]. This restructuring aimed to ensure that each module or class had a single responsibility, thereby adhering to SRP.
Outcomes
The application of SRP led to numerous benefits:
Enhanced Maintainability: The refactored code became more intuitive, simplifying the process of modifying and extending functionalities without affecting unrelated areas[16].
Improved Testability: Testing was made straightforward, as each class was responsi- ble for only one function, reducing the complexity of test cases[17].
Increased Reusability: Classes designed for specific functionalities were more likely to be reused across different parts of the application or in future projects[17].
Better Organization: The overall codebase became better organized, facilitating a more modular approach, which is crucial in modern application development, such as microservices[18].
Transformative Impact of SRP
This case study illustrates the significant positive impact of applying the Single Responsibility Principle within real-world software development. By understanding and implementing SRP, the e-commerce platform team transformed their codebase into a more manageable, scalable, and maintainable system. The approach not only reduced the risk of introducing bugs but also streamlined the development process, allowing for faster iterations and improved overall software quality[15][16].
Benefits of Applying SOLID Principles
Applying the SOLID principles in software development yields numerous advantages that contribute to the overall quality and maintainability of code. These principles serve as a foundation for creating high-quality, robust software systems, and their benefits can be categorized into several key areas.
Improved Maintainability
One of the primary benefits of adhering to SOLID principles is enhanced maintain- ability. By ensuring that each class has a single responsibility (Single Responsibility Principle – SRP), developers can modify specific parts of the codebase without affecting others. This modularity makes it easier to fix bugs and implement new features without introducing unintended side effects[1][19].
Increased Testability
SOLID principles promote better testability of the code. Following the SRP and Inter- face Segregation Principle (ISP) leads to more modular classes, which simplifies the testing process. Developers can create automated tests that verify the functionality of individual components without needing to test the entire system, making it easier to identify and resolve issues early in the development lifecycle[19][20].
Enhanced Extensibility
The Open/Closed Principle (OCP) encourages developers to design software entities that can be extended without modifying their existing code. This flexibility allows for the incorporation of new features or changes in requirements with minimal disruption to the existing codebase. Consequently, applications can evolve over time without requiring extensive rewrites or leading to the introduction of new bugs[21][22].
Consistency and Quality
By applying SOLID principles, developers establish a consistent approach to cod- ing, which promotes uniformity and quality across the codebase. This consistency reduces the cognitive load on team members, enabling them to understand and nav- igate the code more easily, thus leading to better collaboration and decision-making during development[20][22].
Risk Mitigation
Following SOLID principles can significantly reduce the risk of introducing bugs and vulnerabilities into the software. The Dependency Inversion Principle (DIP) and OCP help ensure that changes can be made safely without altering existing, well-tested code. By designing systems that are loosely coupled and highly cohesive, developers can identify and mitigate risks early in the development process[19][22].
Cost-Effectiveness
Implementing SOLID principles can result in cost savings throughout the software development lifecycle. By reducing the incidence of bugs and making maintenance easier, teams can avoid expensive fixes and rework later on. This proactive approach to design ultimately leads to more efficient development processes and a better return on investment for software projects[20][22].
Challenges and Considerations
Interconnected Principles
Many of the SOLID principles are interconnected, meaning that violating one principle can lead to the failure of others. Developers often find it relatively straightforward to adhere to these principles during software design; however, failures commonly arise from haste in implementation or from shortcuts taken during the process.[23] It is essential to invest adequate time in the initial phases of a project, particularly in class and interface design, to avoid potential pitfalls later on.[23]
Application of the Dependency Inversion Principle (DIP)
The Dependency Inversion Principle (DIP) serves as a guideline to enhance appli- cation design and functionality. However, it should not be applied indiscriminately. Experienced developers must assess when and which design principles to employ,
considering the associated costs and efforts in their application.[24] Striking a bal- ance between following guidelines and adapting them to specific contexts is crucial for effective software development.
Common Pitfalls
When implementing the SOLID principles, developers may encounter several com- mon pitfalls:
Over-Segregation
One challenge is over-segregation, where developers create excessively small in- terfaces, resulting in increased complexity. To mitigate this, it is advisable to group closely related methods into cohesive interfaces.[25]
Under-Segregation
Conversely, under-segregation can occur when interfaces are not adequately seg- mented, leading to large, unwieldy interfaces. Regular evaluations of interfaces can help identify unrelated methods that should be separated into more focused interfaces.[25]
Ignoring Client Perspectives
Another potential pitfall involves designing interfaces solely based on technical considerations, neglecting the needs of clients. Engaging with stakeholders to un- derstand their specific requirements is essential for effective interface design.[25]
Tight Coupling Through Interface Inheritance
Developers must also be cautious of tight coupling arising from interface inheritance, which can inadvertently link unrelated functionalities. Such design decisions can complicate the system and lead to maintenance challenges.[25]
Violations of the Liskov Substitution Principle (LSP)
A significant consideration when applying SOLID principles is the Liskov Substitution Principle (LSP). Violating the LSP can result in unexpected behaviors and bugs. An illustrative example is the relationship between birds: while sparrows can fly, penguins cannot. If a penguin is substituted in a context where a flying bird is expected, it leads to a violation of the LSP, demonstrating how improper design choices can break functionality and create inconsistencies in the codebase.[26]
The Importance of Single Responsibility Principle (SRP)
The Single Responsibility Principle (SRP) emphasizes that a class should have one reason to change. Confusion often arises regarding its interpretation, as some may incorrectly equate it with the idea that a class should only perform a single task.
Instead, it should be understood as a class being responsible to one stakeholder or actor, underscoring the need for clarity in design responsibilities.[27]
Team Collaboration and Project Management
Effective collaboration is a cornerstone of successful software development, espe- cially in today’s rapidly evolving technological landscape. True collaboration tran- scends simple task completion, requiring synergy, shared knowledge, and collective problem-solving among team members to achieve common goals.[28] The inte- gration of diverse expertise and perspectives is essential, supported by tools that enhance communication, task management, and project oversight. These tools are particularly vital in remote work settings, where maintaining team cohesion can be challenging due to time zone differences and communication barriers.[28]
The Role of Collaboration in Productivity
Collaboration fosters an environment conducive to innovation and continuous learn- ing. When developers work together, they can leverage their unique skills and insights to tackle complex challenges more effectively than individuals working in isolation.-
[28] This synergy not only accelerates the development process but also enhances the quality of the software produced. Tools tailored for software development, such as version control systems and code review platforms, play a significant role in streamlining workflows and ensuring alignment on project objectives.[28]
Tools for Enhanced Team Collaboration
Utilizing project management software is crucial for distributing tasks among team members, tracking progress, and ensuring timely delivery. Popular platforms like Asana, Microsoft Project, and Zoho Projects offer features that simplify task man- agement and reduce the administrative burden on developers, allowing them to focus on delivering business value.[28][29] Additionally, collaboration tools like Google Workspace and Microsoft Office facilitate real-time document editing and knowledge sharing, which are essential for effective teamwork.[28]
Strategies for Remote Collaboration
For software development teams operating in a remote environment, establishing clear communication protocols is vital for maintaining alignment and productiv- ity. A combination of synchronous and asynchronous communication tools, along with flexible scheduling, can help accommodate team members in different time
zones.[28][30] Furthermore, engaging in virtual team-building activities can cultivate camaraderie and strengthen relationships among team members, enhancing overall team dynamics.
Real-World Benefits of Applying SOLID Principles
- Improved Maintainability: By following SOLID principles, your codebase becomes more modular and easier to understand. When you need to make changes or fix bugs, you can do so with minimal impact on other parts of the system.
- Enhanced Scalability: SOLID principles promote loose coupling between components, making it easier to scale your application. You can add new features or modify existing ones without rewriting large portions of your code.
- Better Testability: SOLID principles, especially SRP and DIP, make your code more testable. You can easily mock dependencies and write unit tests for individual components.
- Flexibility and Extensibility: By adhering to OCP and LSP, your codebase becomes more flexible and easier to extend. You can add new functionality without modifying existing code, reducing the risk of introducing bugs.
- Improved Collaboration: SOLID principles provide a common language and set of best practices for development teams. This leads to more consistent code and easier collaboration among team members.
Conclusion
Applying SOLID principles in real-world scenarios can significantly improve the quality of your codebase. While it may require more upfront design and planning, the long-term benefits in terms of maintainability, scalability, and flexibility are well worth the effort.
Remember, SOLID principles are guidelines, not strict rules. Use them judiciously and always consider the specific needs of your project. As you gain experience, you’ll develop a better intuition for when and how to apply these principles effectively.
By incorporating SOLID principles into your development practices, you’ll be better equipped to create robust, scalable, and maintainable software systems that can stand the test of time and evolving requirements.