Introduction:
Hey there, code wranglers and version control virtuosos! Gather ’round as we embark on a journey deep into the heart of Git, where we’ll uncover some of its most powerful (and dare I say, magical) features. If you’ve been using Git for a while, you might think you’ve got it all figured out. Commit, push, pull, merge – rinse and repeat, right? Well, buckle up, because we’re about to take your Git game to the next level!
In this post, we’re diving headfirst into the world of advanced Git techniques. We’re talking interactive rebasing, cherry-picking, juggling multiple remotes, and more. But this isn’t just a dry tutorial – oh no! We’ll be exploring these concepts through real-world scenarios that’ll make you go, “Aha! So THAT’S how I could’ve solved that git-astrophe last week!”
Whether you’re a solo developer looking to streamline your workflow, or part of a team trying to wrangle a complex project, these advanced Git techniques will give you the superpowers you need to code with confidence. So grab your favorite caffeinated beverage, fire up your terminal, and let’s git (pun totally intended) to it!
A Brief Git Refresher
Before we dive into the advanced stuff, let’s quickly recap the basics. You know, just to make sure we’re all on the same page.
Git, born in 2005 from the brilliant mind of Linus Torvalds (yes, the Linux guy), is a distributed version control system. It’s like a time machine for your code, allowing you to track changes, experiment with new features, and collaborate with others without fear of losing your work.
The basic Git workflow goes something like this:
- You make changes to your files.
- You stage these changes (git add).
- You commit the staged changes (git commit).
- You push your commits to a remote repository (git push).
Simple enough, right? But real-world projects are rarely that straightforward. That’s where our advanced techniques come in handy. So, let’s roll up our sleeves and get into the good stuff!
Interactive Rebasing: Rewriting History (Without a Time Machine)
First up on our magical mystery tour of Git is interactive rebasing. Think of it as editing the director’s cut of your project’s history. With interactive rebasing, you can reorder, edit, squash, or even delete commits before they’re pushed to the shared repository.
The Scenario: Imagine you’re working on a new feature for your app. You’ve made several commits, but looking back, you realize your commit history is a bit… messy. There are typos in commit messages, some commits that should be combined, and maybe even a commit that accidentally included that embarrassing debug print statement. Oops!
The Solution: Interactive Rebasing
Here’s how you’d use interactive rebasing to clean up your history:
git rebase -i HEAD~5
This command will open up your default text editor with the last 5 commits listed, like this:
pick abc1234 Add new login page
pick def5678 Fix typo in login form
pick ghi9101 Add password reset functionality
pick jkl1121 Debug print statement
pick mno3141 Implement password reset email
Now, you can manipulate these commits:
- To fix a commit message, change ‘pick’ to ‘reword’.
- To combine commits, change ‘pick’ to ‘squash’ or ‘s’.
- To delete a commit, simply remove the line.
After saving and closing the file, Git will apply your changes, potentially opening new editor windows for you to modify commit messages.
Pro Tip: Never rebase commits that have been pushed to a shared repository unless you’re absolutely sure no one else has based work on them. Rewriting shared history is a bit like using a time machine to prevent your friends from being born – it can have unintended consequences!
Cherry-Picking: Selecting the Sweetest Commits
Next up is cherry-picking, a technique that allows you to pick specific commits from one branch and apply them to another. It’s like being able to pluck the best ideas from parallel universes and bring them into your own timeline.
The Scenario: You’re working on a long-running feature branch, and you realize that one of your commits contains a critical bug fix that’s needed in the main branch ASAP. You don’t want to merge the entire feature branch yet, but you need that fix in production yesterday.
The Solution: Cherry-Picking
Here’s how you’d cherry-pick that commit:
- First, find the commit hash of the bug fix in your feature branch:
git log feature-branch
- Switch to the branch you want to apply the fix to:
git checkout main
- Cherry-pick the commit:
git cherry-pick abc1234
Git will now apply the changes from that specific commit to your current branch. It’s like magic, but better because it’s real!
Pro Tip: After cherry-picking, always test thoroughly. Sometimes, a commit might have dependencies on other changes in its original branch, which can lead to unexpected behavior when cherry-picked in isolation.
Managing Multiple Remotes: Juggling Code Universes
In the world of open-source and modern development practices, it’s common to work with multiple remote repositories. This could be for collaborating with different teams, contributing to open-source projects, or managing complex deployment pipelines.
The Scenario: You’re working on an open-source project. You have your own fork of the project on GitHub, but you also need to keep your fork updated with the original project’s repository. Plus, you’re experimenting with some changes that you’re not ready to push to your public fork yet, so you have a private repository on GitLab for that.
The Solution: Managing Multiple Remotes
Here’s how you’d set up and manage these remotes:
- View your current remotes:
git remote -v
- Add the original project repository as a remote (commonly called ‘upstream’):
git remote add upstream https://github.com/original/project.git
- Add your private GitLab repository as another remote:
git remote add gitlab https://gitlab.com/your-username/private-project.git
Now you can fetch from and push to these different remotes as needed:
- To update your local main branch with changes from the original project:
git fetch upstream
git checkout main
git merge upstream/main
- To push your experimental changes to your private GitLab repo:
git push gitlab feature-branch
Pro Tip: Use different branch naming conventions for different remotes to keep things organized. For example, ‘upstream/main’ for the original project, ‘origin/feature-x’ for your public fork, and ‘gitlab/experiment-y’ for your private experiments.
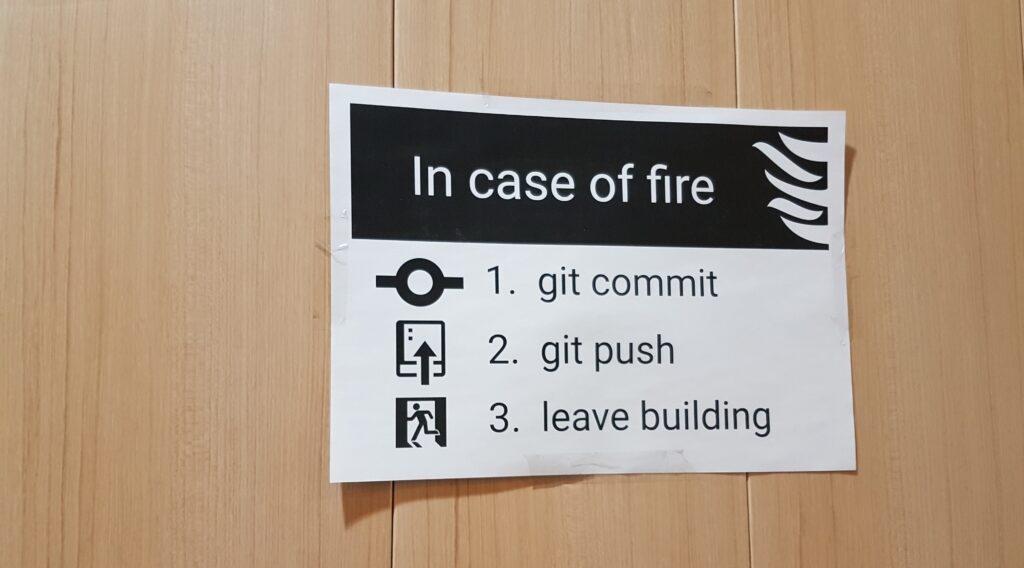
Advanced Merge Strategies: Solving Merge Conflicts Like a Pro
Merge conflicts. Those two words are enough to send shivers down any developer’s spine. But fear not! With advanced merge strategies, you can tackle even the most intimidating merge conflicts with confidence.
The Scenario: You’ve been working on a feature branch for a while. In the meantime, the main branch has seen a lot of activity. Now you need to merge your changes back into main, but Git is screaming about merge conflicts left and right.
The Solution: Advanced Merge Strategies
- The Octopus Merge: When you need to merge more than two branches at once, the octopus merge comes to the rescue:
git merge branch1 branch2 branch3
This creates a single merge commit with multiple parents, which can be cleaner than doing multiple two-way merges. - The Ours Merge: If you want to merge a branch but discard all the changes from that branch, use the ‘ours’ merge strategy:
git merge -s ours obsolete-branch
This is useful for documenting that a branch’s changes were intentionally abandoned. - Interactive Merge with ‘–interactive’: For more control over how changes are applied, use the interactive merge:
git merge --interactive feature-branch
This opens an editor where you can choose which changes to include, similar to interactive rebasing.
Pro Tip: Before attempting a complex merge, create a new branch off your current HEAD. This gives you a safe place to experiment with different merge strategies without affecting your main branch.
Git Hooks: Automating Your Workflow
Git hooks are scripts that Git executes before or after events such as commit, push, and receive. They’re like little robots that can automate parts of your development workflow.
The Scenario: Your team has coding standards that need to be enforced, and you always forget to run the linter before committing. Additionally, you want to make sure that no one accidentally pushes directly to the main branch.
The Solution: Git Hooks
- Create a pre-commit hook to run your linter: In your project’s
.git/hooks
directory, create a file namedpre-commit
:npm run lint
Make it executable:chmod +x .git/hooks/pre-commit
Now, Git will run your linter before every commit, and if it fails, the commit will be aborted. - Create a pre-push hook to prevent direct pushes to main: Create a file named
pre-push
in the same hooks directory:
#!/bin/sh
protected_branch='main'
current_branch=$(git symbolic-ref HEAD | sed -e 's,.*/\(.*\),\1,')
if [ $protected_branch = $current_branch ]
then
echo "Direct push to $protected_branch branch is not allowed. Please use a feature branch and create a pull request."
exit 1
fi
Pro Tip: Store your hooks in your project repository (not in .git/hooks) and create a script to symlink them into .git/hooks. This allows you to version control your hooks and share them with your team.
Submodules and Subtrees: Managing Project Dependencies
For projects that depend on other projects, Git offers two powerful features: submodules and subtrees. These allow you to include other repositories within your main repository.
The Scenario: Your project uses a custom logging library that’s maintained as a separate project. You want to include this library in your main project, keep it up-to-date easily, and possibly contribute back to it.
The Solution: Submodules or Subtrees
- Using Submodules: Submodules allow you to keep a Git repository as a subdirectory of another Git repository. To add a submodule:
git submodule add https://github.com/example/logging-lib.git libs/logging
To update the submodule:git submodule update --remote libs/logging
- Using Subtrees: Subtrees merge the entire history of another project into a subdirectory of your main project. To add a subtree:
git subtree add --prefix libs/logging https://github.com/example/logging-lib.git main --squash
To update the subtree:git subtree pull --prefix libs/logging https://github.com/example/logging-lib.git main --squash
Pro Tip: Submodules are better when you want to keep the projects clearly separated and don’t need to make frequent changes to the included project. Subtrees are better when you want to treat the included project as part of your main project and might need to make changes to it often.
Conclusion: Becoming a Git Wizard
Whew! We’ve covered a lot of ground, haven’t we? From rewriting history with interactive rebasing to managing complex project structures with submodules and subtrees, we’ve explored some of Git’s most powerful features.
Remember, with great power comes great responsibility. These advanced techniques are powerful tools, but they should be used judiciously. Always communicate with your team before using techniques that alter history or change how the repository is structured.
As you start incorporating these advanced Git workflows into your projects, you’ll likely encounter scenarios we haven’t covered here. That’s the beauty of Git – there’s always more to learn, always another way to solve a problem.
So, keep experimenting, keep learning, and most importantly, keep version controlling! Before you know it, you’ll be the one your teammates turn to when they need to untangle a particularly gnarly merge conflict or set up a complex branching strategy.
What’s your favorite advanced Git technique? Have you used any of these in your projects? Share your experiences in the comments below – I’d love to hear about your Git adventures!
And remember, in the immortal words of a Git commit message I once saw: “I have no idea what these changes are supposed to accomplish but Jenkins said I have to commit them.” Here’s to cleaner histories, smoother merges, and fewer mysterious commits in your future!