MongoDB is a popular, open-source NoSQL database that is famous for its scalability and flexibility. It is designed to store large amounts of data in a flexible, JSON-like format called BSON (Binary JSON). Many organizations uses MongoDB because it allows them to easily store, retrieve, and manage large amounts of data in a way that is easy to scale.
MongoDB works by storing data in collections of documents. A collection is a group of related documents, and a document is a set of key-value pairs that represents an entity. Each document can have a different set of fields, and the fields can vary from one document to another. This flexible data model makes it easy to store and query data in a variety of formats and structures.
What we need to learn
To use MongoDB, you will need to learn the basics of the MongoDB query language, namely MongoDB Query Language (MQL). MQL is a powerful and flexible way to query and manipulate data in MongoDB. You will also need to learn how to index and optimize your data for better performance. Additionally, you will need to learn how to work with MongoDB’s sharding and replication features, which allow you to scale your data across multiple servers.
Before you start using MongoDB, it is also important to have a good understanding of database design and data modeling. It will help you avoid common pitfalls and make the most of MongoDB’s features.
MongoDB Query Language (MQL) is the query language we use to manipulate data in MongoDB. It is based on the JSON format and has a number of operators and functions that allow you to query and manipulate data in a variety of ways.
Code Example
For example, suppose you have a collection called “users” that contains documents representing user profiles. Each document has the following fields:
Copy code{
"_id": ObjectId("5f9d1547a89f55a5f5e0b5b5"),
"name": "Alice",
"age": 25,
"email": "alice@example.com"
}
To find all documents in the “users” collection, you can use the following command:
Copy codedb.users.find()
This will return a cursor to all the documents in the collection.
To find documents that match a specific criterion, you can pass a query document to the find()
method. For example, to find all users who are 25 years old, you can use the following command:
Copy codedb.users.find({ age: 25 })
This will return a cursor to all documents in the “users” collection that have an “age” field with a value of 25.
You can also specify multiple criteria in the query document and use various query operators to refine your search. For example, to find all users who are 25 years old and have an email address that ends with “@example.com”, you can use the following command:
Copy codedb.users.find({ age: 25, email: /@example.com$/ })
This will return a cursor to all documents in the “users” collection that have an “age” field with a value of 25 and an “email” field that ends with “@example.com”.
You can also use the find()
method to sort, limit, and skip the returned documents. For example, to find the first 10 users who are 25 years old and sort them by name in ascending order, you can use the following command:
Copy codedb.users.find({ age: 25 }).sort({ name: 1 }).limit(10)
This will return a cursor to the first 10 documents in the “users” collection that have an “age” field with a value of 25, sorted by the “name” field in ascending order.
Mongo Join
MongoDB does not support traditional SQL-style joins like inner join, left join, and right join. Instead, it has a number of ways to query and manipulate data that allow you to achieve the same result as a join in a different way.
One way to perform a join-like operation in MongoDB is to use the $lookup
operator in the aggregate()
pipeline. $lookup
allows you to perform a “left outer join” by combining documents from two collections and returning a new array of documents that contains the joined documents.
Here is an example of how you can use $lookup
to perform a left outer join in MongoDB:
Copy codedb.orders.aggregate([
{
$lookup: {
from: "products",
localField: "productId",
foreignField: "_id",
as: "product"
}
}
])
In this example, the orders
collection contains documents representing orders placed by customers. Each document has a productId
field that references a product in the products
collection. The $lookup
operator combines the documents from the orders
collection with the documents from the products
collection, using the productId
field in the orders
collection as the join key and the _id
field in the products
collection as the foreign key. The result is a new array of documents that contains the joined documents, with the joined documents stored in a field called “product”.
Match Operator
You can also use the $match
operator in the aggregate()
pipeline to filter the joined documents based on certain criteria. For example, to only return orders that have a total price greater than 100, you can use the following pipeline:
Copy codedb.orders.aggregate([
{
$lookup: {
from: "products",
localField: "productId",
foreignField: "_id",
as: "product"
}
},
{
$match: {
"product.price": { $gt: 100 }
}
}
])
This will return a new array of documents that contains only the orders with a total price greater than 100, joined with the corresponding product documents from the products
collection.
You can also use the $merge
operator to perform a left outer join and merge the joined documents into a single collection.
It is worth noting that these methods for performing join-like operations in MongoDB are different from traditional SQL-style joins and may not always be the best solution for your use case. It is important to carefully consider your data model and the type of queries you need to run when deciding how to structure and query your data in MongoDB.
Data Modeling
In MongoDB, it is generally considered best practice to denormalize your data and store related data in a single document or embedded document, rather than using joins to combine data from multiple collections. This is known as “data modeling” in MongoDB.
Data modeling in MongoDB involves designing your data structures and relationships in a way that is optimized for the type of queries and updates you need to perform. It is an important aspect of working with MongoDB and can have a significant impact on the performance and scalability of your application.
Here is an example of how you might define a data model in MongoDB to avoid using joins:
If you have a database that contains information about customer orders and the ordered products, you could use two tables in a traditional relational database to store this information: one for orders and one for products. The two tables would be related to each other through a foreign key. Alternatively, you could use MongoDB to store this data by creating a data model where the orders and products are represented as separate documents in a single collection like this –
Copy code{
"_id": ObjectId("5f9d1547a89f55a5f5e0b5b5"),
"customerId": ObjectId("5f9d1547a89f55a5f5e0b5b6"),
"date": ISODate("2022-01-01T00:00:00Z"),
"totalPrice": 120,
"items": [
{
"productId": ObjectId("5f9d1547a89f55a5f5e0b5b7"),
"name": "Product A",
"price": 100,
"quantity": 1
},
{
"productId": ObjectId("5f9d1547a89f55a5f5e0b5b8"),
"name": "Product B",
"price": 20,
"quantity": 1
}
]
}
Explanation
In this example, the “orders” collection contains a single document per order. The document has fields for the customer id, the date of the order placing, and the total price of the order. It also has an array field called “items” that contains embedded documents representing the individual ordered products. Each product document has a product id, name, price, and quantity.
By storing the product information in the order document itself, you can avoid the need to perform a join to retrieve the product information for a given order. This can improve the performance and scalability of your application, as it reduces the number of operations required to retrieve the data.
It is worth noting that this data model is just one example, and the best data model for your use case will depend on your specific requirements and the type of queries and updates you need to perform. It is important to carefully consider your data model and the type of queries you need to run when deciding how to structure and query your data in MongoDB.
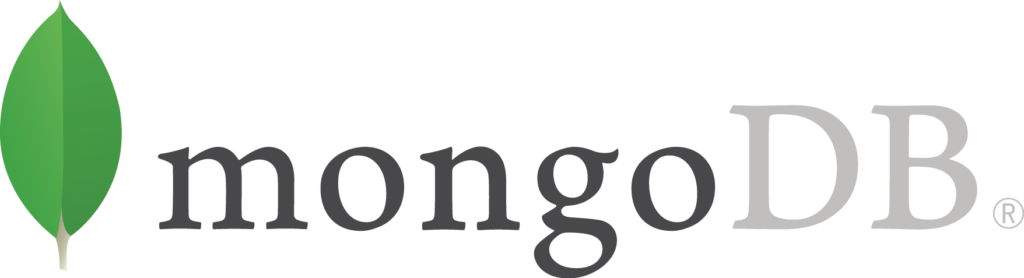
ACID in MongoDB
MongoDB is a NoSQL database and does not support the full ACID (Atomicity, Consistency, Isolation, Durability) properties of a traditional relational database. However, it does provide some mechanisms to ensure the integrity and consistency of data, and it allows you to choose the level of consistency and isolation that is appropriate for your application.
Atomicity: In MongoDB, all write operations on a single document are atomic. This means that if a write operation fails, the entire operation is rolled back and the document is left in its original state. However, MongoDB does not provide atomic write operations across multiple documents.
Consistency: MongoDB uses a concept called “eventual consistency” to ensure the consistency of data. This means that after a write operation is completed, it may take some time for the changes to be reflected in all copies of the data. During this time, different copies of the data may be in different states, but eventually they will all converge to the same state. You can choose the level of consistency you want by using read preferences and write concerns.
Isolation: MongoDB does not support traditional SQL-style transactions, but it does provide some mechanisms to ensure isolation of data. For example, you can use the findAndModify
command to update a document in a collection and return the original document in a single atomic operation. You can also use the $isolated
operator in the update()
command to ensure that updates to a document are not overwritten by concurrent updates.
Durability: MongoDB provides mechanisms to ensure the durability of data, such as write ahead logging and journaling. You can also use replica sets and sharding to provide high availability and data redundancy.
Difference with relational DB
It is worth noting that the ACID properties of MongoDB are not as strong as those of a traditional relational database, and it may not be the best choice for applications that have very strict consistency and isolation requirements. However, for many applications, the flexibility and scalability of MongoDB are more important than the full ACID properties, and the weaker ACID guarantees are sufficient. It is important to carefully consider your consistency and isolation requirements when deciding whether MongoDB is the right choice for your application.
Sharding
Sharding in MongoDB is a way to scale the database horizontally by dividing the data in a collection into smaller chunks called “shards” and distributing them across multiple servers. This is useful when you have a large amount of data and need to distribute the data and workload across multiple servers to improve performance and availability. Each shard is a self-contained unit of data that is stored on a separate server or group of servers.
To shard a collection, you need to choose a shard key, which is a field in the documents of the collection that we can use to determine which shard a document belongs to. The shard key is hashed and the resulting hash is used to map the document to a specific shard.
For example, suppose you have a collection called “users” that contains documents representing user profiles. Each document has the following fields:
Copy code{
"_id": ObjectId("5f9d1547a89f55a5f5e0b5b5"),
"name": "Alice",
"age": 25,
"email": "alice@example.com"
}
To shard the “users” collection, you might choose the “age” field as the shard key. This would allow you to distribute the data in the collection based on the age of the users.
To set up sharding in MongoDB, you need to create a sharded cluster, which consists of a config server, one or more shard servers, and one or more mongos routers. The config server stores metadata about the cluster, including the mapping of documents to shards. The shard servers store the actual data in the shards. The mongos routers route queries and writes to the appropriate shard servers.
Sharding can improve the performance and scalability of your MongoDB deployment by allowing you to distribute the data and workload across multiple servers.
Index in MongoDB
Indexes are special data structures in MongoDB that store a subset of the data in a collection and provide fast access to the data based on the indexed fields. Indexes can improve the performance of queries and updates on a collection by allowing MongoDB to quickly locate the desired data without having to scan the entire collection.
To create an index in MongoDB, you can use the createIndex()
method on the collection. The createIndex()
method takes an index specification document that defines the fields to be indexed and the index options.
Here is an example of how you might create an index on the “name” field of a collection called “users”:
Copy codedb.users.createIndex({ name: 1 })
In this example, the createIndex()
method creates an ascending index on the “name” field. You can also specify a descending index by using a value of -1, or a compound index by specifying multiple fields. For example, to create a compound index on the “name” and “age” fields, you can use the following command:
Copy codedb.users.createIndex({ name: 1, age: 1 })
You can also specify index options like the name of the index, the index type (e.g. “hashed”, “text”, etc.), and the index behavior (e.g. “unique”, “sparse”, etc.) using the createIndex()
method. For example, to create a unique index on the “email” field, you can use the following command:
Copy codedb.users.createIndex({ email: 1 }, { unique: true })
It is important to carefully consider which fields you want to index and how you want to index them. Creating too many indexes can slow down write operations and consume additional disk space, so it is important to strike a balance between performance and efficiency. It is also a good idea to periodically review your indexes to ensure that they are still relevant and necessary for your application.
Key Concept That People Struggle With
There are several concepts that people often struggle with when moving from a relational database to MongoDB. Some of the most common challenges include:
Query language: MongoDB has its own query language called the MongoDB Query Language (MQL), which is different from the Structured Query Language (SQL) used by most relational databases. It can be challenging for people familiar with SQL to learn the syntax and semantics of MQL.
ACID properties: Relational databases support the full ACID (Atomicity, Consistency, Isolation, Durability) properties, which ensure the integrity and consistency of data. MongoDB does not support the full ACID properties and uses a concept called “eventual consistency” to ensure the consistency of data.
Sharding: Sharding is a feature of MongoDB that allows you to scale horizontally by distributing data across multiple servers. It can be challenging for people familiar with single-server databases to understand the concept of sharding and how to design and maintain a sharded MongoDB deployment.
In Summary,
It is worth noting that these challenges are not unique to moving from a relational database to MongoDB, and similar challenges can arise when transitioning to any new database technology. It is important to take the time to learn and understand the concepts and features of the database you are using, and to carefully consider your data model and workload when designing your database schema and queries.
For more details; refer to the official MongoDB Documentation: https://www.mongodb.com/docs/atlas/