Design patterns are a set of best practices and solutions to common software development problems. They provide a way for developers to solve recurring problems in a consistent and efficient manner. In 1994, four software engineers, Erich Gamma, Richard Helm, Ralph Johnson, and John Vlissides, collectively known as the “Gang of Four,” published a book titled “Design Patterns: Elements of Reusable Object-Oriented Software” which introduced 23 design patterns. These patterns have become a fundamental part of the software development industry and are widely used by developers today.
Hey there, fellow code wranglers! Let’s chat about something that’s probably saved my bacon more times than I can count: Design Patterns. Now, I know what you’re thinking – “Oh great, another dry, technical topic.” But stick with me here, because understanding design patterns is like having a secret weapon in your coding arsenal.
I remember when I first stumbled upon design patterns. I was knee-deep in spaghetti code, trying to untangle a mess of a project that had grown way beyond its original scope. It was like playing Jenga with lines of code – one wrong move, and the whole thing would come crashing down. That’s when a senior dev on my team introduced me to the world of design patterns, and let me tell you, it was a game-changer.
These aren’t just abstract concepts dreamed up by ivory tower academics. No, design patterns are battle-tested solutions to problems that developers like you and me face every single day. They’re like recipes for code – tried and true methods that can save you time, headaches, and maybe even your sanity when deadlines are looming.
So, grab your favorite caffeinated beverage, get comfy, and let’s dive into the wonderful world of design patterns. Trust me, your future self will thank you for this!
What are Design Patterns?
Design patterns are reusable solutions to common problems in software development. They provide a way for developers to solve problems in a consistent and efficient manner. They are not specific to any programming language, but rather provide a general solution that can be applied to a variety of languages. Design patterns can be divided into three main categories: creational, structural, and behavioral.
Creational patterns deal with object creation mechanisms, trying to create objects in a manner suitable to the situation. Structural patterns deal with object composition, creating relationships between objects to form larger structures. Behavioral patterns focus on communication between objects, what goes on between objects and how they operate together.
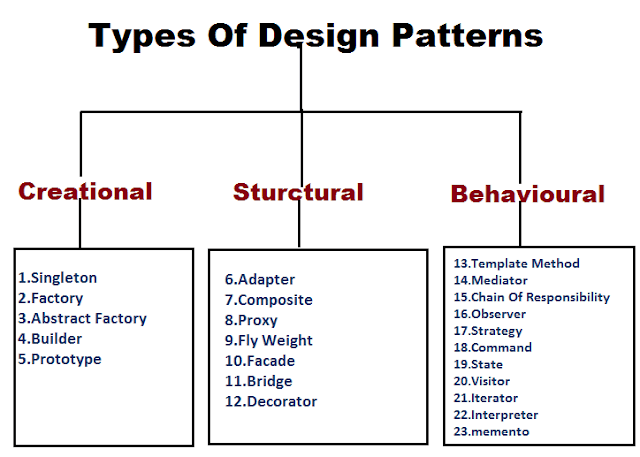
The Gang of Four Patterns
The Gang of Four’s book introduces 23 patterns, which are organized into the three main categories mentioned above. These patterns have become a fundamental part of the software development industry and are widely used by developers today.
- Creational Patterns
- Structural Patterns
- Adapter
- Bridge
- Composite
- Decorator
- Facade
- Flyweight
- Proxy
- Behavioral Patterns
- Chain of Responsibility
- Command
- Interpreter
- Iterator
- Mediator
- Memento
- Observer
- State
- Strategy
- Template Method
- Visitor
Examples of Design Patterns in Action
Design patterns can be used in a variety of situations, and it’s important to understand when to use each one. Here are a few examples of how they can be used in real-world scenarios:
- The Singleton pattern is often used when only one instance of a class is needed throughout the lifetime of an application. This is often the case with objects that manage database connections or configuration settings.
- The Adapter pattern is often used when two incompatible interfaces need to work together. For example, a program written in Java may need to communicate with a legacy system written in C++. The Adapter pattern can be used to create a bridge between the two systems, allowing them to communicate seamlessly.
- The Observer pattern is often used in situations where one object needs to be notified of changes to another object. For example, a weather app may have a subject (WeatherData) that sends updates to multiple observer (CurrentConditionsDisplay, StatisticsDisplay, ForecastDisplay) when the temperature, humidity, or pressure changes.
Now, let’s get real for a second. When I first started using design patterns, I felt like a kid in a candy store. I wanted to use ALL of them, ALL the time. Singleton here, Factory Method there, sprinkle some Observers for good measure… and before I knew it, my codebase looked like a design pattern textbook exploded all over it.
Learn from my mistakes, folks. The key to effectively using design patterns is knowing when to use them – and more importantly, when not to. It’s like seasoning in cooking: a little bit enhances the flavor, but too much ruins the dish.
Tips for you
Here are a few tips I’ve picked up along the way:
- Start simple: Don’t try to force a complex pattern where a simple solution would do. Sometimes, a straightforward approach is all you need.
- Understand the problem first: Before reaching for a design pattern, make sure you fully understand the problem you’re trying to solve. Patterns are tools, not solutions in themselves.
- Consider maintainability: Will other developers (or future you) be able to easily understand and modify the code? Sometimes, a slightly less elegant but more straightforward approach is better in the long run.
- Be prepared to refactor: As your project evolves, you might find that a pattern you initially chose no longer fits. Don’t be afraid to refactor and adapt.
- Communicate with your team: If you’re introducing a new pattern, make sure your team is on board. A pattern that only one person understands can quickly become a maintenance nightmare.
Remember, the goal of using design patterns is to make your code more maintainable, scalable, and easier to understand – not to show off how many patterns you know. Use them wisely, and they’ll be your best friends in the coding trenches.
Exceptions and Special Cases
While design patterns are a great way to solve common problems in software development, it’s important to understand that they are not a one-size-fits-all solution. There may be cases where a design pattern is not the best solution or where it needs to be modified to fit the specific situation. It’s also important to note that design patterns should not be overused, as they can make code more complex and harder to maintain.
Additionally, Design patterns are not a silver bullet and should not be used as a replacement for good software design principles. They should be used in conjunction with other software design principles such as SOLID and DRY.
FAQ
Q: What are Design patterns?
A: Reusable solutions to common problems in software development that provide a way for developers to solve problems in a consistent and efficient manner. They are not specific to any programming language, but rather provide a general solution that can be applied to a variety of languages.
Q: Who are the Gang of Four?
A: Four software engineers, Erich Gamma, Richard Helm, Ralph Johnson, and John Vlissides, who published a book in 1994 titled “Design Patterns: Elements of Reusable Object-Oriented Software” which introduced 23 design patterns. These patterns have become a fundamental part of the software development industry and are widely used by developers today.
Q: What are the three main categories?
A: Creational, structural, and behavioral are the three main categories of design patterns. Creational patterns deal with object creation mechanisms, structural patterns deal with object composition, and behavioral patterns focus on communication between objects.
Q: Are they a one-size-fits-all solution?
A: No, they are not a one-size-fits-all solution. They should be used in conjunction with other software design principles and should not be overused as they can make code more complex and harder to maintain.
Conclusion
Design patterns are a powerful tool for software developers, providing reusable solutions to common problems. The Gang of Four’s book introduced 23 design patterns that have become a fundamental part of the software development industry. Understanding when to use each pattern, and when not to use them, is an essential skill for any software developer. While design patterns are not a one-size-fits-all solution, when used correctly, they can greatly improve the quality and maintainability of software.
Phew! We’ve covered a lot of ground here, haven’t we? From the basics of what design patterns are, to the wisdom of the Gang of Four, all the way to practical tips on using patterns in the real world. But you know what? This is just the tip of the iceberg.
Design patterns are a journey, not a destination. The more you use them, the more you’ll start to see opportunities to apply them in your code. And trust me, there’s nothing quite like that “aha!” moment when you realize a pattern fits perfectly into a problem you’re solving.
Next Step
So, what’s your next step? Well, I’ve got a challenge for you. Pick one pattern we’ve discussed today – maybe the Observer, or the Factory Method, or whichever one caught your eye. Then, try to implement it in a small project. It doesn’t have to be anything fancy – even a simple console application will do.
And hey, why keep all the fun to yourself? Share your experiences in the comments below. Did you have any “aha!” moments? Any frustrations? Any cool ways you adapted a pattern to fit your specific needs? Let’s learn from each other!
Remember, every master was once a beginner. So don’t get discouraged if it feels tough at first. Keep coding, keep learning, and before you know it, you’ll be slinging design patterns like a pro.
Happy coding, and may your patterns always be elegant and your bugs be few!
For more post like this; you may also follow this profile – https://dev.to/asifbuetcse
1 thought on “Design Patterns 101: Understanding the Gang of Four Now”