JavaScript generators is an amazing and brilliant concept; yet it’s very confusing when to use them. They let us write infinite loops that terminate. The yield values from functions before they finish and build lazy data structures. The magic lies in the ability of generators functions to be paused and then resumed again. But before answering ‘how’ and ‘where’; let’s see ‘what’ generator function is.
Introduction
JavaScript generators are a powerful feature introduced in ECMAScript 2015 (ES6) that allow functions to pause and resume execution, yielding multiple values over time. This capability marks a significant advancement in managing asynchronous programming and stateful computations within the JavaScript ecosystem. Generators simplify the complexity associated with traditional asynchronous patterns such as callbacks and promises, enabling developers to write cleaner, more maintainable code that resembles synchronous execution.[1][2]
By adopting the syntax, JavaScript generators utilize the keyword to produce values one at a time, providing a mechanism for lazy evaluation and efficient memory usage. They can significantly enhance the handling of asynchronous operations, allowing for intuitive iteration over potentially large datasets or streaming data, such as paginated API responses.[3][4] Additionally, async generators, introduced alongside this feature, allow for seamless integration with asynchronous data flows, further improving the language’s ability to handle real-time applications.[5][6]
Despite their advantages, the adoption of generators has faced challenges, partic- ularly concerning compatibility across different environments and browsers. While modern browsers support generators extensively, older environments may not, ne- cessitating developers to consider transpilation and polyfills to ensure broader acces- sibility of their applications.[7][8] Furthermore, performance considerations arise, as the stateful nature of generators can introduce overhead, requiring careful evaluation of their use in performance-sensitive contexts.[9]
Overall, JavaScript generators represent a notable evolution in the language’s han- dling of complex asynchronous tasks and iterable data structures, contributing to
a clearer, more efficient coding paradigm. However, developers must navigate the trade-offs associated with compatibility and performance to maximize the benefits of this feature in their projects.[10][11]
Regular generator functions are basically a cross between the Iterator and Observer patterns. A generator is a pause-able function that you can “step” through by calling .next(). We can pull a value out of a generator multiple times with .next(), or push a value into the same function multiple times with .next(valueToPush). This dual interface allows us to imitate both an Iterator and Observer with the same syntax.
Regular functions return only one, single value (or nothing). Generators can return multiple values, one after another, on-demand. They work great with iterables, allowing to create data streams. To create a generator, we need a special syntax construct: function*
. It looks like this:
function* generateSequence() {
yield 'one';
yield 'two';
return 'three';
}
Generator functions behave differently from regular ones. When such function is called, it doesn’t run its code. Instead it returns a special object, called “generator object”, to manage the execution. The main method of a generator is next()
. When called, it runs the execution until the nearest yield<value>
statement. Then the function execution pauses, and the yielded value
is returned to the outer code.
The result of next()
is always an object with two properties:
value
: the yielded value.done
:true
if the function code has finished, otherwisefalse
.
function* generateSequence() {
yield 'one';
yield 'two';
return 'three';
}
let generator = generateSequence();
let output1 = generator.next();
alert(JSON.stringify(output1)); // {value: 'one', done: false}
let output2 = generator.next();
alert(JSON.stringify(output2)); // {value: 'two', done: false}
let output3 = generator.next();
alert(JSON.stringify(output3)); // {value: 'three', done: true}
alert(JSON.stringify(output3)); // {value: undefined, done: true}
Now let’s turn our focus on ‘where’ –
- Lets say we want to play and pause loops if someone hit a pause button on an animation. Generators can be used with this for loops which need to be paused and resumed at a later time.
- We want an infinitely looping array for things like carousels or lists of data which wrap around. Generators can be used for infinitely looping over an array and having it reset to the beginning once it’s done.
- We want to iterate over objects to check if any of its properties include a specific value. We use generators by creating iterables to use in ‘for of ‘ loops from non-iterable objects using [Symbol.Iterator]; get the array and then check with
.includes()
. - to loop through structures where we’d normally need to keep track of multiple variables at a time.
Let’s see some ‘how’ of these ‘where’.
#1: looping arrays to pause and resume
function* makeGeneratorLoop(arr) {
for (const value of arr) {
yield value;
}
}
const genExample = makeGeneratorLoop([0, 1, 9, 8, 6, 2]);
console.log(genExample.next());
#2: Infinitely looping array
function* makeGeneratorLoop(arr) {
for (let i = 0;; i++) {
if (i === arr.length) i = 0;
yield arr[i];
}
}
const genExample = makeGeneratorLoop([0, 1, 9, 8, 6, 2]);
console.log(genExample.next());
#3: Iterable from Object
const myObj = {
name: 'Asif',
Job: 'Developer',
age: 27
}
myObj[Symbol.iterator] = function* () {
for (const prop in this) {
yield this[prop];
}
}
console.log([...myObj]); // > ["Asif", "Developer", 27]
for (const val of myObj) {
console.log(val)
}
Javascript Generators Use Case?
In a normal function, there is only one entry point – the invocation of the function itself. A generator allows us to pause the execution of a function and resume it later. Generators are useful when dealing with iterators and can simplify the asynchronous nature of JavaScript.
generators are a really powerful tool that facilitates cleaner code — especially when it comes to any kind of async behavior. A side benefit of generators is easier testing. For example, we could test a program by writing a different interpreter, that asserts the yielded commands without executing them.
How does yield work JavaScript?
The yield keyword pauses generator function execution and retains the value of the expression after the yield keyword is returned to the generator’s caller. yield can only be called directly from the generator function that contains it. It cannot be called from nested functions or from callbacks. Once paused on a yield expression, the generator’s code execution remains paused until the generator’s next() method is called. Each time the generator’s next() method is called, the generator resumes execution.
What other cases you have used javascript generators? Let us know by commenting down below!
Generators are iterable
generators are iterable. We can loop over their values using for..of
:
function* generateSequence() {
yield 1;
yield 2;
return 3;
}
let generator = generateSequence();
for(let value of generator) {
alert(value); // 1, then 2
}
Looks a lot nicer than calling .next().value
, right?
But please note: the example above shows 1
, then 2
, and that’s all. It doesn’t show 3
!
It’s because for..of
iteration ignores the last value
, when done: true
. So, if we want all results to be shown by for..of
, we must return them with yield
:
function* generateSequence() {
yield 1;
yield 2;
yield 3;
}
let generator = generateSequence();
for(let value of generator) {
alert(value); // 1, then 2, then 3
}
As generators are iterable, we can call all related functionality, e.g. the spread syntax ...
:
function* generateSequence() {
yield 1;
yield 2;
yield 3;
}
let sequence = [0, ...generateSequence()];
alert(sequence); // 0, 1, 2, 3
In the code above, ...generateSequence()
turns the iterable generator object into an array of items.
Generator composition
Generator composition is a special feature of generators that allows to transparently “embed” generators in each other.
For instance, we have a function that generates a sequence of numbers:
function* generateSequence(start, end) {
for (let i = start; i <= end; i++) yield i;
}
Now we’d like to reuse it to generate a more complex sequence:
- first, digits
0..9
(with character codes 48…57), - followed by uppercase alphabet letters
A..Z
(character codes 65…90) - followed by lowercase alphabet letters
a..z
(character codes 97…122)
We can use this sequence e.g. to create passwords by selecting characters from it (could add syntax characters as well), but let’s generate it first.
In a regular function, to combine results from multiple other functions, we call them, store the results, and then join at the end.
For generators, there’s a special yield*
syntax to “embed” (compose) one generator into another.
The composed generator:
function* generateSequence(start, end) {
for (let i = start; i <= end; i++) yield i;
}
function* generatePasswordCodes() {
// 0..9
yield* generateSequence(48, 57);
// A..Z
yield* generateSequence(65, 90);
// a..z
yield* generateSequence(97, 122);
}
let str = '';
for(let code of generatePasswordCodes()) {
str += String.fromCharCode(code);
}
alert(str); // 0..9A..Za..z
The yield*
directive delegates the execution to another generator. This term means that yield* gen
iterates over the generator gen
and transparently forwards its yields outside. As if the values were yielded by the outer generator.
The result is the same as if we inlined the code from nested generators:
function* generateSequence(start, end) {
for (let i = start; i <= end; i++) yield i;
}
function* generateAlphaNum() {
// yield* generateSequence(48, 57);
for (let i = 48; i <= 57; i++) yield i;
// yield* generateSequence(65, 90);
for (let i = 65; i <= 90; i++) yield i;
// yield* generateSequence(97, 122);
for (let i = 97; i <= 122; i++) yield i;
}
let str = '';
for(let code of generateAlphaNum()) {
str += String.fromCharCode(code);
}
alert(str); // 0..9A..Za..z
A generator composition is a natural way to insert a flow of one generator into another. It doesn’t use extra memory to store intermediate results.
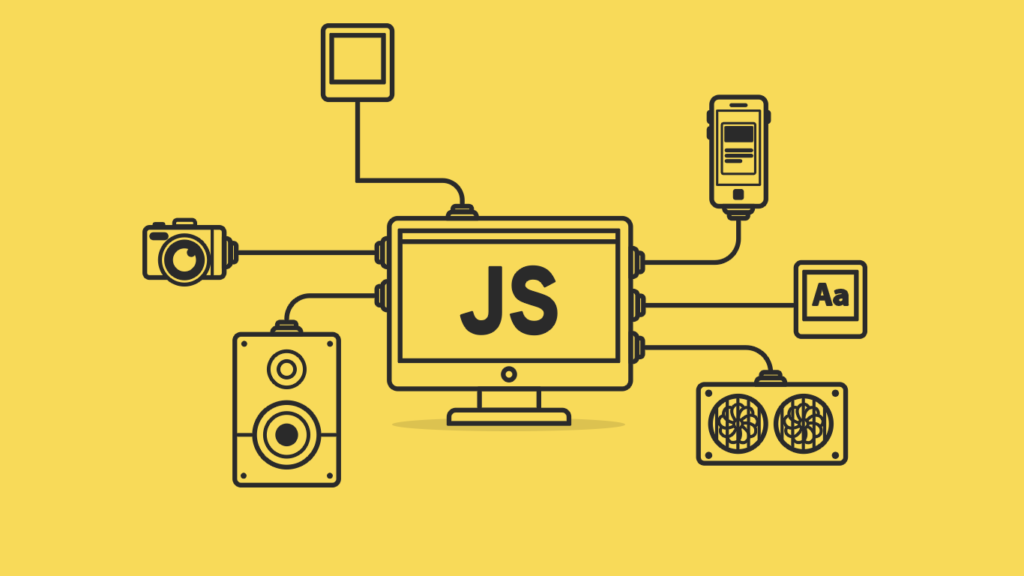
“yield” is a two-way street
Until this moment, generators were similar to iterable objects, with a special syntax to generate values. But in fact they are much more powerful and flexible. That’s because yield
is a two-way street: it not only returns the result to the outside, but also can pass the value inside the generator. To do so, we should call generator.next(arg)
, with an argument. That argument becomes the result of yield
.
Let’s see an example:
function* gen() {
// Pass a question to the outer code and wait for an answer
let result = yield "2 + 2 = ?"; // (*)
alert(result);
}
let generator = gen();
let question = generator.next().value; // <-- yield returns the value
generator.next(4); // --> pass the result into the generator
- The first call
generator.next()
should be always made without an argument (the argument is ignored if passed). It starts the execution and returns the result of the firstyield "2+2=?"
. At this point the generator pauses the execution, while staying on the line(*)
. - Then, as shown at the picture above, the result of
yield
gets into thequestion
variable in the calling code. - On
generator.next(4)
, the generator resumes, and4
gets in as the result:let result = 4
.
Please note, the outer code does not have to immediately call next(4)
. It may take time. That’s not a problem: the generator will wait.
For instance:
// resume the generator after some time
setTimeout(() => generator.next(4), 1000);
As we can see, unlike regular functions, a generator and the calling code can exchange results by passing values in next/yield
.
To make things more obvious, here’s another example, with more calls:
function* gen() {
let ask1 = yield "2 + 2 = ?";
alert(ask1); // 4
let ask2 = yield "3 * 3 = ?"
alert(ask2); // 9
}
let generator = gen();
alert( generator.next().value ); // "2 + 2 = ?"
alert( generator.next(4).value ); // "3 * 3 = ?"
alert( generator.next(9).done ); // true
- The first
.next()
starts the execution… It reaches the firstyield
. - The result is returned to the outer code.
- The second
.next(4)
passes4
back to the generator as the result of the firstyield
, and resumes the execution. - …It reaches the second
yield
, that becomes the result of the generator call. - The third
next(9)
passes9
into the generator as the result of the secondyield
and resumes the execution that reaches the end of the function, sodone: true
.
It’s like a “ping-pong” game. Each next(value)
(excluding the first one) passes a value into the generator, that becomes the result of the current yield
, and then gets back the result of the next yield
.
generator.throw
As we observed in the examples above, the outer code may pass a value into the generator, as the result of yield
.
…But it can also initiate (throw) an error there. That’s natural, as an error is a kind of result.
To pass an error into a yield
, we should call generator.throw(err)
. In that case, the err
is thrown in the line with that yield
.
For instance, here the yield of "2 + 2 = ?"
leads to an error:
function* gen() {
try {
let result = yield "2 + 2 = ?"; // (1)
alert("The execution does not reach here, because the exception is thrown above");
} catch(e) {
alert(e); // shows the error
}
}
let generator = gen();
let question = generator.next().value;
generator.throw(new Error("The answer is not found in my database")); // (2)
The error, thrown into the generator at line (2)
leads to an exception in line (1)
with yield
. In the example above, try..catch
catches it and shows it.
If we don’t catch it, then just like any exception, it “falls out” the generator into the calling code.
The current line of the calling code is the line with generator.throw
, labelled as (2)
. So we can catch it here, like this:
function* generate() {
let result = yield "2 + 2 = ?"; // Error in this line
}
let generator = generate();
let question = generator.next().value;
try {
generator.throw(new Error("The answer is not found in my database"));
} catch(e) {
alert(e); // shows the error
}
If we don’t catch the error there, then, as usual, it falls through to the outer calling code (if any) and, if uncaught, kills the script.
History
JavaScript generators were introduced as part of ECMAScript 6 (ES6), which rep- resented one of the most significant updates to the language’s core functionality. The concept of generators allows functions to yield multiple values over time, en- abling a more efficient way of handling asynchronous programming and stateful computations. This feature marked a shift towards improving JavaScript’s handling of asynchronous operations, an area that had been evolving through various patterns, including callbacks and promises[1][2].
Before generators became part of the ECMAScript specification, developers often relied on traditional function calls to manage asynchronous processes. This led to complex and sometimes unwieldy code structures, especially when nesting multiple asynchronous operations. Generators provide a syntactically cleaner approach, al- lowing developers to pause and resume function execution, which simplifies the flow of asynchronous code[3][4].
The design and implementation of generators were influenced by existing concepts in other programming languages, such as Python’s generators, which had already proven effective for similar use cases. As a result, JavaScript’s generators adopted the syntax, allowing functions to yield values using the keyword, a pattern that was new to JavaScript at the time of its introduction[1][5].
Despite the advantages of using generators, their adoption has encountered chal- lenges, particularly regarding browser support. While modern browsers widely sup- port generators, developers targeting older environments have faced compatibility issues. This necessitates careful consideration of the target audience when imple- menting generators in JavaScript applications[2][4].
Syntax
JavaScript generators are a special type of function that can pause and resume their execution. They are defined using the syntax, where the asterisk (*) indicates that the function is a generator[6][7]. Within a generator function, the keyword is utilized to yield values one at a time, allowing the function to pause its execution until the next value is requested via the method[7][8].
Defining a Generator Function
A generator function can be declared as follows:
In this example, the function yields the values 1 and 2 before returning 3. However, when iterating over the generator using a loop, only the yielded values (1 and 2) are accessible, as the return value (3) is not included in the iteration[6][1].
Using Generators
To create a generator object from a generator function, the function is invoked like any other function. The generator’s state can be controlled using the `.
After all values are yielded, further calls to will indicate that the generator is done, returning [7].
Async Generators
In addition to standard generators, JavaScript also supports asynchronous generator functions, which can be declared using the syntax.
This approach enhances the functionality of generators by integrating them seam- lessly with asynchronous data streams, allowing for more intuitive handling of data in real-time applications[7][9].
Usage
JavaScript generators are a powerful feature that allows for the creation of iterable sequences and the handling of asynchronous data flows. Their utility can be broken down into several practical applications.
Handling Asynchronous Data
Generators can be particularly effective for managing asynchronous operations. For example, using async generators simplifies the process of working with paginated data from APIs. When retrieving large datasets, such as a list of products from an
e-commerce API, an async generator allows developers to fetch data page by page without the complexity of chaining promises or using recursion. This results in cleaner, more maintainable code[10].
Simplifying Complex Data Flows
In scenarios where multiple asynchronous events need to be processed over time, async generators provide a structured approach. By yielding data as it becomes available, developers can write code that resembles synchronous iteration, making it easier to follow and debug. For instance, when fetching user data, an async generator can yield each user object as it is received, allowing for immediate processing without waiting for the entire dataset[10].
Iteration with Custom Objects
JavaScript generators can also be used to add iteration capabilities to custom objects. By implementing the method, objects can be made iterable, enabling the use of the loop to traverse through the object’s properties or values. This is particularly useful in scenarios where you need to work with non-array data structures in a familiar iteration format[11].
Project Implementation
When setting up a project that incorporates JavaScript generators, it’s essential to establish a structured environment. For example, developers can start by creating a project folder, initializing it with their preferred package manager, and structuring the application to separate the main code from the generator logic. This modular
approach facilitates the management of complex applications where generators play a critical role in handling asynchronous data and simplifying iteration[12].
Comparison with Other Features
JavaScript generators provide a unique mechanism for handling asynchronous pro- gramming and data iteration, distinguishing themselves from traditional functions and other language features.
Yielding Values
One of the key differences between generators and standard functions is the use of the keyword, which allows generators to pause their execution and yield values to the caller. When combined with the syntax, generators can delegate yielding to another generator or iterable, enabling more complex iterations.[13] For instance, can return a value upon completion, which behaves differently from a typical return in functions, allowing the entire generator to evaluate to the returned value from the associated generator function.[13]
Iterators and Built-in Iterables
Generators are closely related to the concept of iterators. An iterator in JavaScript implements the iterable protocol, providing a method that returns objects containing and properties.[14][15] Unlike standard iterators, which are often used in loops or for array manipulations, generators simplify the creation of iterators by allowing devel- opers to define the sequence of values using the syntax. Furthermore, generators can iterate over built-in iterable objects like arrays, strings, and maps, enhancing their versatility in handling diverse data structures.[13]
Asynchronous Programming
In addition to their iterator capabilities, generators also play a significant role in asynchronous programming. While traditional asynchronous patterns often rely on callbacks or promises, generators allow for a more synchronous-like flow using the
keyword. This makes it easier to manage sequences of asynchronous operations, albeit through the use of helper functions to handle the generator’s execution.[16]
Performance Considerations
From a performance perspective, generators can introduce some overhead due to their stateful nature and the need to maintain the execution context. This can be contrasted with more straightforward asynchronous approaches like callbacks or promises, which may have lower latency in certain scenarios. However, the ease
of managing complex flows and maintaining code readability often outweighs these concerns in applications where maintainability and clarity are prioritized.[12]
Conceptual Connections
When utilizing generators, it is beneficial to connect concepts through practical applications. As one practices with generators, implementing related projects can reinforce understanding and reveal potential use cases beyond mere iterations, encouraging a deeper exploration of the language’s capabilities.[5]
Examples
Basic Generator Function
A generator function is defined using the syntax, which indicates that the function can yield multiple values over time.
In this example, the execution is paused after yielding , and can be resumed later with the value of being set to 6 before the function completes its execution[17][18].
Generating Sequences
Generators can be used to produce a sequence of values, which can be particularly useful in iteration contexts.
This generator can be used with the loop to produce each value in the specified range sequentially, demonstrating the efficiency and readability of using generators[11][19].
Real-life Example: Paginated Data
Generators can also be applied to real-world scenarios, such as handling paginated data from APIs. For example, when fetching commits from a GitHub repository, the API returns a limited number of results per request, alongside a URL for the next page of results.
In this example, the generator fetches and yields each commit as it is retrieved, simplifying the handling of potentially large datasets[20][19].
Utility Functions with Generators
Generators are also helpful for creating utility functions that can generate unique identifiers or handle complex logic without blocking execution. For example, they can generate all possible moves in a game or seek particular values among permutations and combinations[9][21].
By leveraging generators, developers can build more robust and efficient applications that maintain clarity and simplicity in their code structures.
Performance
JavaScript generators provide several performance advantages, particularly in terms of memory efficiency and execution speed in specific use cases. Generators are special types of iterator objects that can yield multiple values over time, allowing for lazy evaluation, which can significantly reduce memory usage compared to traditional arrays[14][22].
Memory Efficiency
One of the primary benefits of using generators is their ability to handle large datasets without loading all values into memory at once. Generators produce values on demand, meaning they only hold one value in memory at any given time. This
is particularly advantageous for memory-intensive collections, as it enables efficient management of resources. For instance, if a generator is implemented to generate a range of numbers, it will only retain the current number in memory rather than storing the entire range in an array, which would require substantially more memory[23][14].
Execution Speed
While the performance difference between generators and traditional iterators can vary based on implementation and specific use cases, generators can lead to faster execution in scenarios where only a subset of values is needed at any one time. Since generators allow the code to pause and resume execution, they can help to optimize processes that involve asynchronous programming patterns, such as those found in JavaScript’s event-driven model[24][22].
However, it is essential to note that the choice between using generators and other techniques should be based on clarity and maintainability of code, as performance optimization might not always justify the added complexity. Developers are encour- aged to measure performance and apply optimizations judiciously, focusing on areas where actual bottlenecks are identified through proper performance profiling[25][26].
Error Handling
Error handling in JavaScript is an essential aspect of developing robust applications, particularly when working with generators. Generators, which are functions that can be paused and resumed, introduce unique considerations for error management that developers must understand to effectively debug and maintain their code.
The Role of Error Handling in Generators
Error handling in generators is similar to traditional error handling in JavaScript, yet it also encompasses additional mechanisms that allow for more nuanced control flows. When an error occurs within a generator, it can be caught and handled using the block, providing a way to manage exceptions that may arise during the execution of the generator’s code[27][28].
Using Try-Catch Blocks
Developers can encapsulate code within a block and respond to any thrown errors in the corresponding block. This structure is particularly useful for handling asynchro- nous operations or operations that depend on external inputs.
In this example, if an error is thrown during the execution of the generator, it will be caught and logged, demonstrating how to effectively handle errors within generator functions[29][30].
Throwing Errors in Generators
In addition to catching errors, generators can also throw errors explicitly using the method. This capability allows developers to communicate specific issues that may arise during execution.
If a developer needs to indicate an error, they can use the method at any point within the generator, and it will be caught in the next iteration[31][32].
Best Practices for Error Handling in Generators
Descriptive Error Messages: Providing clear and meaningful error messages is vital. This practice helps in diagnosing issues quickly, especially when dealing with complex generator logic[33].
Using Finally Blocks: Incorporating a block within your generator can ensure that necessary cleanup code runs regardless of whether an error was thrown or not. This is particularly useful for releasing resources or resetting states[28].
Handling State Carefully: Generators maintain their state between calls, and develop- ers should be cautious when pausing and resuming execution. It is crucial to ensure that the state remains consistent, especially when errors can alter the expected flow[32][30].
Compatibility
JavaScript generators are a powerful feature introduced in ECMAScript 2015 (ES6) that allow for the creation of iterator objects using the syntax. Their compatibility varies across different environments and browsers, necessitating awareness for developers aiming to leverage this feature.
Browser Support
Most modern browsers provide robust support for generators. Google Chrome, Mozil- la Firefox, Microsoft Edge, and Safari have implemented generator functions since their early versions following the ES6 specification. As of now, all major browsers support generators without any significant issues, allowing developers to use this feature confidently in production environments[12].
Node.js Compatibility
In the Node.js environment, generators have been supported since version 0.11.0, which introduced experimental support for the feature. By the time Node.js reached version 4.0.0, generators were fully integrated and usable without flags. As of current versions, Node.js continues to maintain full compatibility with generator functions, making them a reliable choice for server-side JavaScript applications[34][35].
Transpilation and Polyfills
For projects that aim to support older browsers that do not implement generator functionality, developers often use tools like Babel, which transpile ES6 code into ES5 code. This allows for the conversion of generator functions into compatible constructs, albeit sometimes with a performance trade-off. Developers can also utilize polyfills to mimic generator behavior in environments lacking native support, though this may come at the cost of increased complexity and performance[4].
Use Cases and Considerations
Given their capabilities, such as lazy evaluation and asynchronous programming, generators are particularly useful in scenarios involving large datasets or complex asynchronous workflows. However, developers should evaluate the specific require- ments of their applications and the environments they target to determine if the use of generators is appropriate, balancing performance and compatibility needs[36].
Libraries and Frameworks
JavaScript generators can be effectively utilized within various libraries and frame- works that enhance their functionality and ease of use in applications.
Data Grid Libraries
Kendo UI
Kendo UI is a comprehensive library that offers a data grid with live data loading capabilities across different frameworks including jQuery, Angular, Vue, and React. It features column and row virtualization for optimized performance and provides
extensive customization options through themes. Kendo UI is not open-source and comes with various support services for licensed users[35].
DevExtreme Data Grid
Part of the DevExtreme component suite, the DevExtreme Data Grid includes es- sential features like filtering, sorting, grouping, and searching. It provides robust API documentation and a range of live demos, facilitating effective learning and implementation for developers[35].
Handsontable
Handsontable is a spreadsheet-like data grid that boasts a plethora of features, including custom column headers, summaries, and extensive filtering and sorting options. It supports various cell types and offers capabilities such as clipboard functionality, exporting to multiple file formats, and virtual rendering for improved per- formance. Handsontable integrates seamlessly with popular frameworks like Angular, React, and Vue, making it a versatile choice for data-heavy applications[35].
These libraries not only enhance the usability of JavaScript generators but also offer developers powerful tools for managing complex data operations efficiently. By leveraging these libraries, developers can build applications that are not only robust but also optimized for performance, ensuring a seamless user experience.