Event handling is a critical aspect of web development, and it’s important to have a good understanding of how events work in order to build robust and maintainable applications. In this conversation, we explored the concepts of event listeners in ASP.NET Web Forms, including the event handler signature, why they are declared as protected, and the relevant concepts of event handling. We also discussed the importance of event validation and event delegation in the context of event listeners in ASP.NET Web Forms.
Introduction
ASP.NET Web Forms Event Handling is a vital feature of the ASP.NET framework that facilitates the development of interactive web applications through an event-driven programming model. This model allows developers to create dynamic user interfaces by responding to various events triggered by user interactions, such as button clicks or form submissions. As web applications have become increasingly complex and user-centric, the importance of a robust event handling mechanism has grown, making it a notable aspect of ASP.NET Web Forms development. The framework’s approach to event handling is similar to that of traditional desktop applications, making it accessible for developers familiar with event-driven paradigms. The event handling system in ASP.NET Web Forms is built around a structured page life cycle that includes multiple stages—initialization, loading, and unloading—during which various events are raised. This structure enables developers to manage application behavior effectively, allowing for the execution of custom code at specific points in the page life cycle. Additionally, ASP.NET supports automatic event wire-up, which simplifies the binding of event handlers to events, thus reducing boilerplate code and enhancing developer productivity. Such features contribute to the framework’s popularity in rapidly developing enterprise-level applications where user interactivity is paramount. However, the ASP.NET Web Forms event handling model is not without its controversies and challenges. Critics often point out that the reliance on ViewState for maintaining control states across postbacks can lead to performance issues, particularly in larger applications, due to increased page size. Moreover, the complexity of managing cascading event handlers can result in unpredictable behaviors, underscoring the necessity for best practices in event management. Developers are encouraged to implement strategies such as event delegation and centralized event handling to enhance maintainability and performance. Overall, the event handling mechanism in ASP.NET Web Forms plays a crucial role in the framework’s functionality, allowing for the creation of responsive applications while also presenting challenges that developers must navigate to optimize performance and maintainability. By leveraging the event-driven model effectively, developers can create sophisticated web applications that meet modern user expectations for interactivity and responsiveness.
Signature
In ASP.NET Web Forms, an event listener typically has the following signature:
protected void EventName_Event(object sender, EventArgs e)
where “EventName” is the name of the event being listened for and “Event” is the type of the event (e.g. “Click”, “SelectedIndexChanged”). The “sender” parameter represents the object that raised the event, and the “e” parameter contains information about the event.
Event listeners are declared as “protected” because they are intended to be used only by the class or control that defines them. Making them protected prevents them from being accessed or overridden by external classes or code. Additionally, it’s a best practice to make them protected, because the event listener is part of the implementation of the class, and should not be exposed to external consumers of the class.
Why listeners are not declared private?
Listeners could be declared as private, however it would limit the ability to subclass and override the behavior of the listener. By declaring the listener protected, it allows the class that defines the listener to handle the event, as well as any subclass that may inherit from it to also handle the event and potentially override the behavior.
Additionally, some events may be intended to be handled by other controls or classes that are part of the same application, so making the listener private would make it impossible for those other controls or classes to handle the event.
It’s worth noting that in some cases it may be appropriate to make an event listener private, if the listener is intended for internal use only and should not be overridden or accessed by external code. However, in general, making event listeners protected is a best practice because it allows for flexibility in handling the event and for the ability for subclasses to customize the behavior.
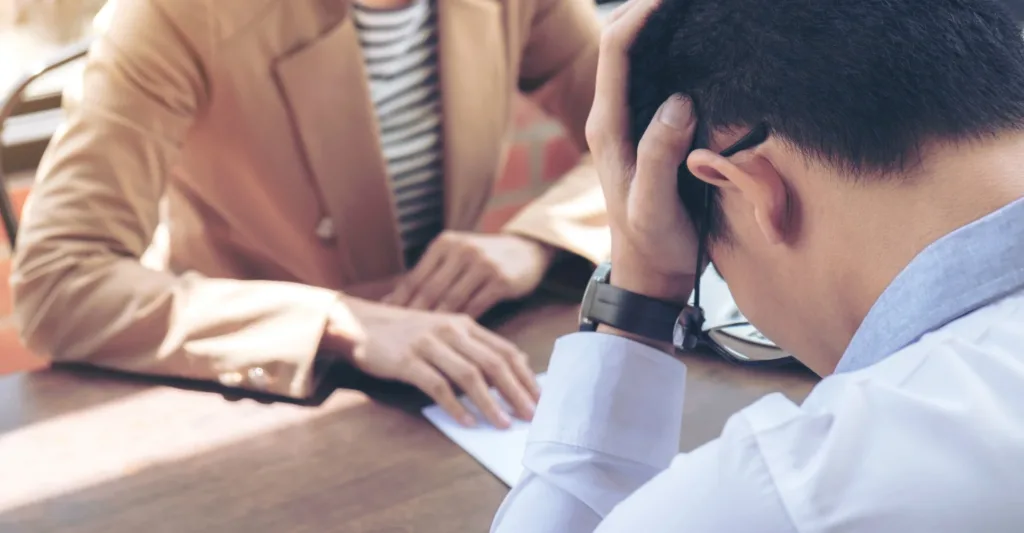
why listeners are not declared public? why they are declared void?
Listeners are not declared public because they are intended to be used only within the class or control that defines them, and not by external classes or code. Public event listeners would be accessible to any code in the application, which could lead to unintended consequences or security issues.
Listeners are declared void because they do not return a value. They are intended to perform some action in response to the event, such as updating the state of the control or updating the user interface.
While the standard signature for an event listener in ASP.NET Web Forms is protected void EventName_Event(object sender, EventArgs e)
, it is possible to declare an event listener with a different signature, depending on the requirements of your application. For example, you could declare a listener with a different return type or with different parameters. However, it’s important to keep in mind that the standard signature is used by the framework and that using a different signature may make it difficult to interact with the framework’s built-in functionality.
Another way to declare an event listener is using the EventHandler<TEventArgs>
delegate, where TEventArgs is a custom class that you can use to pass more information along with the event. This way, the signature of the event listener will be like EventName += new EventHandler<TEventArgs>(EventName_Event);
Keep in mind that whatever the way you choose to declare the event listener, it should be consistent with the .NET framework conventions, otherwise it may lead to confusion for developers who read and maintain your code.
Explain event validation in context of event listener in dot net web form.
Event validation is a security feature in ASP.NET Web Forms that is used to ensure that events are only raised by controls that are part of the current page. This helps to prevent security vulnerabilities such as cross-site scripting (XSS) attacks.
When a web page is rendered, the ASP.NET framework generates a unique validation key for each control on the page. This validation key is included as a hidden field in the HTML for the page, and is also included as a value in the HTML for each control that can raise events.
When a user interacts with a control and an event is raised, the client-side JavaScript in the browser sends a request to the server along with the validation key for the control that raised the event. The ASP.NET framework then compares the validation key sent in the request with the validation keys for the controls on the current page. If the validation key sent in the request does not match a validation key for a control on the current page, the event is not processed and an exception is thrown.
Event validation is enabled by default in ASP.NET Web Forms, and can be disabled by setting the EnableEventValidation
property to false
in the Page
directive or in the web.config file. However, it is not recommended to disable event validation as it can lead to a security vulnerability on your application.
Event validation is important because it ensures that events are only raised by controls that are part of the current page, and not by controls that have been added to the page by an attacker. This helps to prevent security vulnerabilities such as cross-site scripting (XSS) attacks.
Explain event Delegation in context of event listener in dot net web form
Event delegation is a technique in ASP.NET Web Forms that allows you to assign a single event handler to handle multiple events in the same way. This can be useful when you have multiple controls on a page that should perform the same action when an event is raised.
For example, you may have a page with several buttons, and you want to handle the click event for all of them in the same way. Instead of creating a separate event handler for each button, you can use event delegation to assign a single event handler to all of the buttons.
Event delegation is implemented by using the +=
operator to add a single event handler to multiple controls. Here is an example:
button1.Click += new EventHandler(Button_Click);
button2.Click += new EventHandler(Button_Click);
button3.Click += new EventHandler(Button_Click);
In this example, the Button_Click
event handler is assigned to the Click
event of three buttons. When any of the buttons is clicked, the Button_Click
event handler is executed.
You can also use the -=
operator to remove the event handler from the control.
Event delegation can help you to keep your code more organized and maintainable. It allows you to centralize the event handling logic for multiple controls in a single event handler, instead of having to create separate event handlers for each control. This can make your code easier to read and understand, and make it easier to make changes in the future.
It’s worth noting that Event delegation also allows you to handle events from controls that are added dynamically to the page, and also to handle events from a parent container control that propagates the event from a child control.
Event Model
In ASP.NET Web Forms, the event model is a crucial aspect that allows developers to create interactive and responsive web applications. It is based on an event-driven programming paradigm, where the flow of the application is determined by events, such as user interactions or system notifications. This approach enables components to communicate with one another in a loosely coupled manner, enhancing the maintainability and scalability of applications[1][2].
Overview of Event Handling
Events in ASP.NET are notifications sent by objects when certain actions occur, such as user actions or state changes. Developers can create event handlers to respond to these events, allowing for customized behavior in web applications. For example, a button control can trigger a click event, to which a developer can bind a specific action to execute when the button is clicked[3][1]. The concept of events in ASP.NET is similar to the event handling found in desktop application development, where each application state is treated as an object that can change in response to events[3][2].
Page Life Cycle Events
The ASP.NET page life cycle involves multiple stages during which various events are raised. Each time a page is requested, it goes through initialization, processing, and disposal stages, raising events that developers can handle to run custom code. This includes automatic event wire-up, where ASP.NET binds certain method names to corresponding page events, facilitating event handling without manual intervention[- 3][4]. For instance, methods like and are automatically invoked when the respective events are raised, provided the attribute is set to true[4].
Creating and Raising Events
Developers define custom events using delegates and the event keyword in C#.
Here, is a delegate type that specifies the method signature for the event handler, and is the event itself. To raise the event, the developer typically checks certain conditions and calls the event if they are met, allowing other parts of the application to subscribe and respond to the event[5][2].
Best Practices for Event Usage
To effectively utilize events within applications, several best practices should be con- sidered. These include avoiding cascading event handlers, which can lead to complex and unpredictable behavior, and implementing event aggregators to centralize event management. Additionally, batching multiple rapid events into a single event can help reduce performance overhead, ensuring the application remains responsive[2][6].
By adhering to these practices, developers can leverage the full potential of the event-driven model in ASP.NET Web Forms.
Event Handling Mechanism
In ASP.NET Web Forms, the event handling mechanism is a fundamental aspect that enables developers to create interactive applications by responding to user actions and system events. This model is predominantly event-based, allowing for seamless integration of event-driven programming principles within the framework.
Understanding Events
An event in ASP.NET serves as a notification system, allowing a class to inform its clients when significant occurrences or state changes happen within an object.
Events are commonly recognized in user interfaces, where actions such as button clicks or text changes trigger corresponding notifications[1]. They act as signals for specific actions that need to be executed, effectively guiding the flow of application logic.
Page Life Cycle and Event Handling
The life cycle of an ASP.NET page involves several key stages, during which various events are raised. These stages include initialization, control instantiation, state maintenance, event handling, and rendering[3]. Each time a page is requested, it is
processed through these stages, allowing developers to handle events accordingly. Notably, the ASP.NET runtime performs these steps each time the page is called, ensuring that events can be managed at the appropriate moments within the cycle[4].
Event Binding
Developers can bind event handlers to events declaratively using attributes in the markup, such as , or programmatically in the code-behind files[4]. This flexibility allows for both simple and complex interactions within the user interface, making it easier to create dynamic web applications.
Automatic Event Wire-up
ASP.NET also supports automatic event wire-up, which simplifies event handling by allowing methods to be automatically bound to events based on naming conventions. For instance, when the attribute is set to true in the directive, methods named ac- cording to the pattern (e.g., , ) are automatically executed when their corresponding events are raised. This feature significantly reduces the amount of boilerplate code required for event handling, streamlining the development process[4].
Common Events
ASP.NET Web Forms utilize a robust event-driven model that allows developers to respond to user interactions and system changes effectively. Events in ASP.NET are crucial for enabling dynamic user interfaces and maintaining a responsive application environment.
Overview of Events in ASP.NET
Events in ASP.NET Web Forms are similar to events in other programming environ- ments, functioning as notifications that indicate that a specific action has occurred. For example, a button click can trigger an event that executes a corresponding event handler method, allowing the application to react accordingly. Each event is tied to a specific control on the page, such as buttons, text boxes, and other user interface elements[2][6].
Types of Common Events
User Interface Events
User interface events are among the most prevalent in ASP.NET applications. They allow developers to handle user interactions seamlessly.
Click Event: Triggered when a user clicks on a button. For instance, when a button is clicked, an event handler can be executed to perform an action, such as displaying a message box or submitting a form.
Mouse Events: Events like MouseOver and MouseOut allow for further interaction based on mouse movements, enhancing the user experience.
Page Life Cycle Events
Every ASP.NET page goes through a well-defined life cycle, during which various events are raised that developers can hook into to execute custom code.
Page_Init: This event occurs early in the page life cycle and is ideal for initializing page properties and controls.
Page_Load: Triggered after the page is initialized, this event is typically used to load data and perform actions every time the page is requested.
Page_Unload: This event is fired when the page is about to be removed from memory, allowing developers to release resources or perform cleanup operations[3][4].
Automatic Event Wiring
ASP.NET simplifies event handling with automatic event wire-up, which binds specific methods to events based on naming conventions. For example, if the AutoEven- tWireup attribute is set to true, methods like are automatically associated with the Page’s Load event, streamlining the development process[4].
Practical Examples
Event Handling in User Controls
In ASP.NET Web Forms, event handling can be effectively demonstrated through user controls that communicate with each other. A common scenario involves a source user control that sends a message and a destination user control that receives it.
To facilitate this interaction, developers create custom events and utilize delegates. For example, a source user control may contain a button that, when clicked, raises a custom event. This event can then carry additional information via a custom event argument class that inherits from , enabling the message to be passed along to the destination user control[7][6].
Example: Button Click Event
A straightforward example of event handling can be observed with button controls. In a typical scenario, when a button is dragged and dropped onto a Windows Form, a click event handler is automatically generated upon double-clicking the button in the design view. This event handler is responsible for responding to user interactions.
This code snippet showcases how multiple objects can subscribe to the same event, allowing them to react when the event is triggered[6][8]. The parameter contains information about the button that was clicked, while provides additional data relevant to the event.
Centralized Event Management
To manage events effectively, developers often implement an event aggregator pattern. This pattern centralizes event handling, reducing direct interactions between individual components, which helps to decouple the components and improve main- tainability. By using an event aggregator, multiple components can subscribe to the same events without requiring direct references to each other, facilitating a more modular design[2][9].
Handling Multiple Events
When a single method needs to handle multiple button click events, the keyword can be employed in ASP.NET. This approach simplifies event management by allowing one method to respond to multiple controls.
In this example, the method serves as a handler for click events from three different buttons. The method uses the parameter to identify which button was clicked, showcasing a flexible and reusable event handling strategy[8][10].
Best Practices
Understanding Event Handling
Effective event handling is crucial in ASP.NET Web Forms to create responsive and maintainable applications. Developers should be familiar with the standard event handler signature, typically defined as , where indicates the control that triggered the event, and provides event-specific information[11]. Following established con- ventions aids in code readability and maintenance.
Implementing Event Delegation
Event delegation is a valuable technique that allows developers to manage events more efficiently. By assigning a single event handler to multiple controls, the code- base becomes more organized and easier to maintain[11]. This is particularly useful for dynamically added controls, as it allows for centralized handling of events without the need for separate handlers for each control.
Security Considerations
Event validation is a critical security feature in ASP.NET Web Forms that ensures events are raised only by legitimate controls on the current page. This mechanism helps prevent vulnerabilities such as cross-site scripting (XSS) attacks[11][12]. De- velopers should ensure that event validation is not disabled, as doing so can expose the application to security risks.
Naming Conventions for Asynchronous Methods
When creating asynchronous methods, it is recommended to follow a naming con- vention that appends “Async” to the original method name (e.g., ). This clarity improves the code’s usability and maintains consistency across the application[13]. Additionally, implementing asynchronous operations for long-latency tasks enhances user experience by keeping the application responsive.
Continuous Learning and Community Engagement
Developers should stay informed about the latest trends and best practices in state management and event handling through community forums, official documentation, and online courses. Engaging with the ASP.NET community can provide insights into innovative techniques and frameworks that enhance application performance and maintainability[14].
Practical Steps for Implementation
Build a Portfolio: Showcase projects that highlight the effective use of event handling and state management strategies.
Understand Trade-Offs: Be aware of the performance and scalability implications of different event handling approaches, ensuring that the chosen method aligns with application requirements[14].
Prioritize Security: Always adopt secure coding practices, ensuring that event han- dling logic does not expose vulnerabilities within the application[14].
By following these best practices, developers can enhance the quality of their ASP.NET Web Forms applications, creating robust, maintainable, and secure solu- tions.
ViewState and Event Handling
ViewState is a fundamental component of ASP.NET Web Forms that aids in manag- ing the state of controls across postbacks. It operates by storing the state of controls in a hidden field on the page, which is sent back and forth between the client and server during each postback. This mechanism ensures that values entered by users in forms, such as text boxes and checkboxes, are retained even after the page is submitted[15][16]. However, it is important to note that ViewState is limited to the page it resides on, meaning it does not share data across different pages[17].
Mechanism of ViewState
ViewState functions automatically within the ASP.NET framework, saving control state during the page lifecycle and restoring it during postbacks[17]. This process allows for a seamless user experience by preserving control values without requiring explicit handling by developers. Despite its utility, developers must be aware of the potential performance penalties associated with ViewState, especially for data-heavy pages, as it increases the size of the web page and can impact load times[16][18].
Event Handling in Postbacks
When a user interacts with a web page, such as clicking a button, a postback event is triggered, sending the current state of the page to the server for processing. During this process, the IsPostBack property helps determine whether the page is being loaded for the first time or as a result of a postback[19]. This is crucial for executing code conditionally during the initial page load, ensuring that state is maintained appropriately.
Event handlers in the code-behind file are responsible for managing specif-
ic user-triggered events, such as Button_Click or DropDownList_SelectedIndex- Changed. These handlers contain the logic to be executed when the corresponding event occurs, allowing developers to manipulate control states and perform actions based on user interactions[19][11].
Challenges and Best Practices
While ViewState simplifies state management, it also poses certain challenges, including potential performance bottlenecks due to increased page load times and security risks if not properly managed. To mitigate these issues, developers are encouraged to minimize the use of ViewState for controls that do not require state persistence, instead opting for alternative mechanisms like Session State or Cache when appropriate[19][18]. Additionally, employing AJAX techniques for partial page updates can enhance performance by reducing the need for full postbacks, thus improving the overall user experience[19][20].
Asynchronous Event Handling
Overview of Asynchronous Operations
In ASP.NET Web Forms, asynchronous operations can enhance the user experience by allowing long-running tasks to be executed without blocking the user interface.
Implementing asynchronous functionality is particularly useful when operations may incur noticeable delays, such as network requests or file I/O[13]. This allows the application to remain responsive even under high user loads.
Event-based Asynchronous Pattern (EAP)
The Event-based Asynchronous Pattern (EAP) provides a standardized approach for implementing asynchronous features in a class. By following this pattern, developers can define events to report the progress of asynchronous operations, using a object that carries an integer progress indicator ranging from 0 to 100[13]. A common practice is to name these events according to the operation they represent, such as or , to maintain clarity[13].
Implementing EAP in ASP.NET Web Forms
When implementing the EAP in an ASP.NET Web Forms application, developers can utilize helper classes like to ensure compatibility across different application models, including Console applications and Windows Forms applications. This implementa- tion allows for notifying clients upon the completion of operations or when significant progress is made[13].
Best Practices for Asynchronous Event Handling
Naming Conventions: Define an asynchronous counterpart for each synchronous method by appending to the method name, such as . This asynchronous method should return and accept the same parameters as its synchronous counterpart. Optionally, an overload with an additional parameter can be defined[13].
Cancellation Support: Optionally, implement cancellation features to allow clients to cancel ongoing operations gracefully. This enhances user control and experience[- 13].
Progress Reporting: Provide support for progress reporting by raising events that notify clients of updates during the operation[13]. This can be particularly useful in long-running tasks where users may benefit from knowing the status.
Incremental Results: Consider implementing support for returning incremental re- sults, which allows clients to receive data in stages rather than waiting for the entire operation to complete[13].
Considerations for Asynchronous Operations
While asynchronous programming offers significant advantages, it is crucial to im- plement it judiciously. Excessive use of asynchronous operations can lead to thread exhaustion and reduced performance. Developers should carefully evaluate when to apply the EAP based on the specific needs of their applications and the expected user interactions[21][22]. By leveraging the EAP effectively, ASP.NET Web Forms applications can deliver a more efficient and responsive user experience.
Best Practices
Understanding Event Handling
Effective event handling is crucial in ASP.NET Web Forms to create responsive and maintainable applications. Developers should be familiar with the standard event handler signature, typically defined as , where indicates the control that triggered the event, and provides event-specific information[11]. Following established con- ventions aids in code readability and maintenance.
Implementing Event Delegation
Event delegation is a valuable technique that allows developers to manage events more efficiently. By assigning a single event handler to multiple controls, the code-
base becomes more organized and easier to maintain[11]. This is particularly useful for dynamically added controls, as it allows for centralized handling of events without the need for separate handlers for each control.
Security Considerations
Event validation is a critical security feature in ASP.NET Web Forms that ensures events are raised only by legitimate controls on the current page. This mechanism helps prevent vulnerabilities such as cross-site scripting (XSS) attacks[11][12]. De- velopers should ensure that event validation is not disabled, as doing so can expose the application to security risks.
Naming Conventions for Asynchronous Methods
When creating asynchronous methods, it is recommended to follow a naming con- vention that appends “Async” to the original method name (e.g., ). This clarity improves the code’s usability and maintains consistency across the application[13]. Additionally, implementing asynchronous operations for long-latency tasks enhances user experience by keeping the application responsive.
Continuous Learning and Community Engagement
Developers should stay informed about the latest trends and best practices in state management and event handling through community forums, official documentation, and online courses. Engaging with the ASP.NET community can provide insights into innovative techniques and frameworks that enhance application performance and maintainability[14].
Practical Steps for Implementation
Build a Portfolio: Showcase projects that highlight the effective use of event handling and state management strategies.
Understand Trade-Offs: Be aware of the performance and scalability implications of different event handling approaches, ensuring that the chosen method aligns with application requirements[14].
Prioritize Security: Always adopt secure coding practices, ensuring that event han- dling logic does not expose vulnerabilities within the application[14].
By following these best practices, developers can enhance the quality of their ASP.NET Web Forms applications, creating robust, maintainable, and secure solu- tions.
Comparison with Other Frameworks
ASP.NET Web Forms and ASP.NET MVC are two distinct frameworks within the ASP.NET ecosystem, each serving different development needs. While they share
some underlying technology, they differ significantly in architecture and programming paradigms.
Architectural Differences
Web Forms employs an event-driven programming model, enabling developers to create web applications using a drag-and-drop interface similar to WinForms applications. This approach allows for rapid application development but can lead to challenges in managing state and complexity as applications grow[21][23]. In contrast, ASP.NET MVC follows the Model-View-Controller (MVC) design pattern,
promoting a clear separation of concerns. This structure allows developers to manage complexity by dividing the application into distinct components: the model (data), the view (UI), and the controller (business logic) [24][25].
State Management
One key difference between the two frameworks is how they handle state. Web Forms relies heavily on view state and server-side controls, making state manage- ment more straightforward for simple applications. However, this reliance can lead to increased page size and slower performance as applications scale[26][25]. In contrast, ASP.NET MVC does not use view state, which provides developers with greater control over application behavior and can lead to more optimized and efficient code[27][24].
Testing and Maintenance
Testing and maintainability are critical considerations when choosing a framework. Web Forms can be challenging to test due to its reliance on event handlers that are fired only in specific web contexts[9]. On the other hand, ASP.NET MVC facilitates better unit testing and code maintenance, as its architecture encourages more modular design and separation of concerns, making it easier to isolate components for testing[28][27].
Developer Experience and Suitability
The choice between Web Forms and MVC often depends on the development team’s expertise and project requirements. Web Forms is generally considered more accessible for developers with less experience, thanks to its visual designer and component-based approach, which speeds up the development process for smaller teams[26][21]. Conversely, ASP.NET MVC is favored by developers who prefer a structured, code-centric approach and need the flexibility to work with modern web technologies like HTML5, Ajax, and Web APIs[24][29].
Ultimately, while ASP.NET MVC is not a direct replacement for Web Forms, it provides a more modern framework that aligns better with contemporary web development practices. The decision to use one framework over the other should be guided by the specific needs of the project and the preferred development style of the team[28][27]..
Conclusion
Event handling is a key component of web development, and mastering it is essential to building robust and maintainable applications. In this conversation, we looked at the concepts of event listeners in ASP.NET Web Forms, including their signature, why they are declared as protected, and how event validation and event delegation are used to improve the security and maintainability of your application. With this information, you should have a better understanding of how events work in ASP.NET Web Forms and be better equipped to build web applications that are both secure and easy to maintain.
For more post like this; you may also follow this profile – https://dev.to/asifbuetcse